如何在Python中实现图的数据结构并使用邻接表进行存储,然后再进行图的深度优先遍历
时间: 2024-11-15 19:33:52 浏览: 8
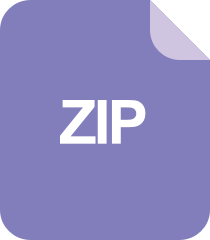
基于python实现深度优先遍历搜索(DFS).zip
在Python中,可以使用内置的数据结构如列表或字典来实现图数据结构,并利用邻接表的方式进行存储。邻接表是一种常用表示图的方式,它包含两个主要部分:
1. **节点(Vertices)**: 表示图中的每个元素,通常是一个列表或者字典,其中键是节点本身,值是与其相连的边的集合。
2. **边(Edges)**: 使用邻接表,对于每一个节点,其对应的值就是一个列表,包含了连接该节点的所有其他节点。
下面是一个简单的邻接表实现:
```python
class Graph:
def __init__(self):
self.vertices = {} # 使用字典作为邻接表
def add_vertex(self, vertex):
if vertex not in self.vertices:
self.vertices[vertex] = []
def add_edge(self, vertex1, vertex2):
if vertex1 in self.vertices and vertex2 in self.vertices:
self.vertices[vertex1].append(vertex2)
self.vertices[vertex2].append(vertex1) # 邻接表通常是无向的,所以双向添加
# 使用示例
g = Graph()
g.add_vertex('A')
g.add_vertex('B')
g.add_vertex('C')
g.add_edge('A', 'B')
g.add_edge('B', 'C')
def depth_first_search(graph, start_vertex):
visited = set() # 记录已访问过的节点
stack = [start_vertex] # 深度优先搜索堆栈
while stack:
vertex = stack.pop() # 取出下一个未访问的节点
if vertex not in visited:
visited.add(vertex) # 标记节点为已访问
print(vertex, end=' ') # 输出节点
for neighbor in graph.vertices[vertex]: # 探索邻居
stack.append(neighbor)
# 进行深度优先遍历
depth_first_search(g, 'A') # 结果可能是 "A B C"
```
阅读全文
相关推荐
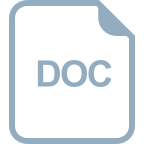
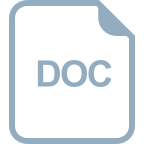
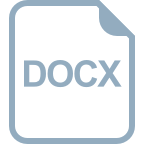
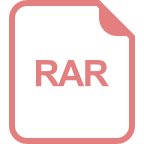
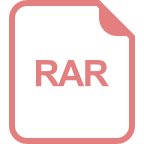
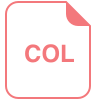











