使用C语言生成一个随机迷宫
时间: 2024-09-21 14:03:42 浏览: 82
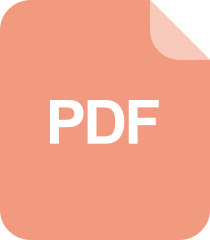
C++随机生成迷宫算法
在C语言中创建一个随机迷宫通常涉及到二维数组或矩阵的使用,以及一些基本的随机数生成。这里是一个简单的基于深度优先搜索(DFS)和回溯算法的示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
#define ROWS 10 // 迷宫的行数
#define COLS 10 // 迷宫的列数
typedef struct {
int maze[ROWS][COLS];
int visited[ROWS][COLS]; // 记录是否访问过的位置
} Maze;
void create_maze(Maze *maze, int start_row, int start_col) {
srand(time(NULL)); // 设置随机种子
maze->maze[start_row][start_col] = 0; // 起点标记为0表示通路
maze->visited[start_row][start_col] = 1;
// DFS方向选择(上下左右)
directions[] = {0, 1, 0, -1};
int direction = rand() % 4;
while (true) {
int next_row = start_row + directions[direction];
int next_col = start_col + directions[(direction+1)%4];
if (next_row >= 0 && next_row < ROWS &&
next_col >= 0 && next_col < COLS &&
maze->maze[next_row][next_col] == 1 && // 避免进入已填的墙壁
!maze->visited[next_row][next_col]) {
maze->maze[next_row][next_col] = 0; // 创建通路
maze->visited[next_row][next_col] = 1;
// 检查当前位置是否有未探索的方向
for (int i = 0; i < 4; i++) {
int new_direction = (i + direction + 4) % 4;
int newRow = next_row + directions[new_direction];
int newCol = next_col + directions[(new_direction + 1) % 4];
if (!maze->visited[newRow][newCol]) {
start_row = newRow;
start_col = newCol;
break;
}
}
if (maze->visited[start_row][start_col]) {
break; // 找到出口,结束回溯
}
} else {
// 回溯到上一步
maze->maze[start_row][start_col] = 1; // 将路径变为墙壁
maze->visited[start_row][start_col] = 0;
direction = (direction + 1) % 4;
}
}
}
// 显示迷宫
void display_maze(Maze *maze) {
for (int i = 0; i < ROWS; i++) {
for (int j = 0; j < COLS; j++) {
printf("%d ", maze->maze[i][j]);
}
printf("\n");
}
}
int main() {
Maze maze;
create_maze(&maze, 0, 0);
display_maze(&maze);
return 0;
}
```
这个程序首先初始化一个迷宫和访问标志,然后从起点开始进行深度优先搜索,创建通路并记录已经访问过的区域。最后,它会打印出生成的迷宫。
阅读全文
相关推荐
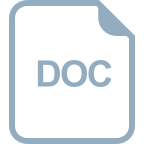
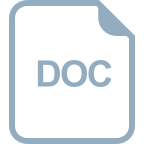












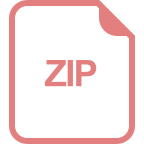