condition=(df2['current_height']>200||df2['current_height']<25) rows_to_drop=df2[condition] df2=df2.drop(rows_to_drop.index)
时间: 2024-04-12 09:28:07 浏览: 110
在 Python 中,`||` 并不是逻辑或的运算符,而是位运算符。在逻辑运算中,我们应该使用 `or` 关键字代替 `||`。此外,要同时满足两个条件,你应该使用 `&` 来进行逻辑与运算。以下是修改后的代码:
```python
condition = (df2['current_height'] > 200) | (df2['current_height'] < 25) # 使用 or 和逻辑与运算符 &
rows_to_drop = df2[condition]
df2 = df2.drop(rows_to_drop.index)
```
请注意,代码中使用了 `|` 来表示逻辑或运算,`&` 来表示逻辑与运算。这样,`condition` 就会筛选出满足条件的行,并使用 `drop()` 方法删除这些行。
相关问题
帮我解释一下错误:UnicodeDecodeError Traceback (most recent call last) Cell In[4], line 3 1 import pandas as pd 2 df1 = pd.read_csv('beijing_wangjing_125_sorted.csv') ----> 3 df2 = pd.read_csv('D:\Users\Downloads\07-机器学习入门\望京LINE.csv') 4 merged_df = pd.merge(df1, df2, left_on='id', right_on='ID') 5 merged_df.to_csv('merged.csv', index=False) File ~\anaconda3\lib\site-packages\pandas\util_decorators.py:211, in deprecate_kwarg.<locals>._deprecate_kwarg.<locals>.wrapper(*args, **kwargs) 209 else: 210 kwargs[new_arg_name] = new_arg_value --> 211 return func(*args, **kwargs) File ~\anaconda3\lib\site-packages\pandas\util_decorators.py:331, in deprecate_nonkeyword_arguments.<locals>.decorate.<locals>.wrapper(*args, **kwargs) 325 if len(args) > num_allow_args: 326 warnings.warn( 327 msg.format(arguments=_format_argument_list(allow_args)), 328 FutureWarning, 329 stacklevel=find_stack_level(), 330 ) --> 331 return func(*args, **kwargs) File ~\anaconda3\lib\site-packages\pandas\io\parsers\readers.py:950, in read_csv(filepath_or_buffer, sep, delimiter, header, names, index_col, usecols, squeeze, prefix, mangle_dupe_cols, dtype, engine, converters, true_values, false_values, skipinitialspace, skiprows, skipfooter, nrows, na_values, keep_default_na, na_filter, verbose, skip_blank_lines, parse_dates, infer_datetime_format, keep_date_col, date_parser, dayfirst, cache_dates, iterator, chunksize, compression, thousands, decimal, lineterminator, quotechar, quoting, doublequote, escapechar, comment, encoding, encoding_errors, dialect, error_bad_lines, warn_bad_lines, on_bad_lines, delim_whitespace, low_memory, memory_map, float_precision, storage_options) 935 kwds_defaults = _refine_defaults_read( 936 dialect, 937 delimiter, (...) 946 defaults={"delimiter": ","}, 947 ) 948 kwds.update(kwds_defaults) --> 950 return _read(filepath_or_buffer, kwds) File ~\anaconda3\lib\site-packages\
这个错误是 `UnicodeDecodeError`,意味着在读取 CSV 文件时遇到了无法解码的 Unicode 字符。
在 Pandas 中,`read_csv` 函数默认使用 UTF-8 编码读取 CSV 文件。如果文件使用其他编码(例如 GBK、GBK2312 等)保存,就需要在读取时指定正确的编码。你可以使用 `encoding` 参数来指定编码,例如:
```python
df2 = pd.read_csv('D:\Users\Downloads\07-机器学习入门\望京LINE.csv', encoding='GBK')
```
如果你不确定文件的编码,可以尝试使用 Python 标准库中的 `chardet` 模块来自动检测编码。你可以使用以下代码来检测文件的编码:
```python
import chardet
with open('D:\Users\Downloads\07-机器学习入门\望京LINE.csv', 'rb') as f:
result = chardet.detect(f.read())
print(result['encoding'])
```
这段代码会输出文件的编码,你可以将其作为 `encoding` 参数的值来读取文件。
另外,如果文件中确实存在无法解码的字符,你可以尝试使用 `errors` 参数来指定处理方式。例如,你可以使用 `errors='ignore'` 忽略无法解码的字符,或者使用 `errors='replace'` 将无法解码的字符替换为问号。
对以下代码进行讲解:df1 = pd.read_excel('附件2(Attachment 2)2023-51MCM-Problem B.xlsx', index_col=None) df2 = pd.read_excel('附件3(Attachment 3)2023-51MCM-Problem B.xlsx', index_col=None) df1_grouped = df1.groupby(['日期(年/月/日) (Date Y/M/D)','发货城市 (Delivering city)', '收货城市 (Receiving city)'])['快递运输数量(件) (Express delivery quantity (PCS))'].sum().reset_index() df2_grouped = df2.groupby(['起点 (Start)'])['终点 (End)'] cities = set(df2['起点 (Start)'].tolist() + df2['终点 (End)'].tolist()) workbook = load_workbook(filename="附件3(Attachment 3)2023-51MCM-Problem B.xlsx") sheet = workbook.active data = [[cell.value for cell in row[:3]] for row in sheet.iter_rows(min_row=2)] date = '2023-04-23' df1_date = df1[df1['日期(年/月/日) (Date Y/M/D)'] == date] data1 = list(zip(df1_date['发货城市 (Delivering city)'], df1_date['收货城市 (Receiving city)'], df1_date['快递运输数量(件) (Express delivery quantity (PCS))'])) G=nx.DiGraph() G.add_nodes_from(cities) G.add_weighted_edges_from(data) cost=0 for i in data1: start_city=i[0] end_city=i[1] weight_huo=i[2] print(dijkstra_path(G,start_city,end_city,weight="weight")) print(dijkstra_path_length(G,start_city,end_city,weight="weight")*(1+ (weight_huo/200)*(weight_huo/200)*(weight_huo/200))) cost=cost+dijkstra_path_length(G,start_city,end_city,weight="weight")*(1+ (weight_huo/200)*(weight_huo/200)*(weight_huo/200)) print(cost)
这段代码主要是对两个Excel文件中的数据进行处理,并使用Dijkstra算法计算货物运输的成本。
首先,代码通过`pd.read_excel`函数读取了两个Excel文件,分别是'附件2(Attachment 2)2023-51MCM-Problem B.xlsx'和'附件3(Attachment 3)2023-51MCM-Problem B.xlsx'。`index_col=None`参数表示不使用任何列作为索引。
接下来,使用`groupby`函数对第一个Excel文件进行分组操作。按照'日期(年/月/日) (Date Y/M/D)'、'发货城市 (Delivering city)'和'收货城市 (Receiving city)'这三列进行分组,并计算'快递运输数量(件) (Express delivery quantity (PCS))'列的总和。结果保存在`df1_grouped`中。
同样地,对第二个Excel文件进行分组操作,按照'起点 (Start)'这一列进行分组。结果保存在`df2_grouped`中。
接下来,通过将第二个Excel文件中的'起点 (Start)'和'终点 (End)'列合并,得到所有的城市,并将其保存在`cities`集合中。这里使用`set`函数将两列的数据转换为集合,并使用`tolist`函数将集合转换为列表。
然后,使用`load_workbook`函数加载第二个Excel文件,并选择其中的活动工作表。通过遍历工作表的行和列,将数据存储在二维列表`data`中。对于每行数据,通过列表推导式将单元格的值提取出来。
接下来,定义了一个日期变量`date`,用于筛选第一个Excel文件中指定日期的数据。
然后,通过筛选出指定日期的数据,将发货城市、收货城市和快递运输数量存储在名为`data1`的列表中。这里使用`zip`函数将三个列的数据打包成元组,并使用`list`函数将结果转换为列表。
接下来,创建一个有向图对象`G`,并根据第二个Excel文件中的数据添加节点和带权重的边。使用`add_nodes_from`方法将城市添加为节点,使用`add_weighted_edges_from`方法将起点、终点和权重添加为边。
然后,定义了一个变量`cost`用于存储成本,并通过遍历`data1`中的每个元组进行计算。对于每个元组,依次提取出起始城市、目标城市和货物重量。
在循环中,首先通过调用`dijkstra_path`函数计算从起始城市到目标城市的最短路径,并打印出结果。然后,通过调用`dijkstra_path_length`函数计算最短路径的长度,并根据货物重量计算成本。这里成本的计算方式是:路径长度乘以一个系数(1 + (weight_huo/200)^3)。
最后,将每次循环计算得到的成本累加到`cost`变量中,并打印出最终的成本。
总体来说,这段代码主要是对两个Excel文件中的数据进行处理,并使用Dijkstra算法计算货物运输的成本。
阅读全文
相关推荐
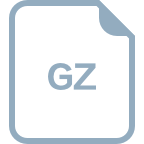
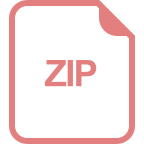
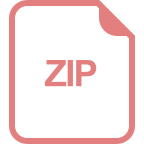













