使用遗传算法计算并输出 f(x,y) 最大值。 适应度函数: f(x,y)= (6.452(x+0.1225y)(cos(x)−cos(2y)) ²) /(0.8+(x−4.2) ² +2∗(y−7)² ) +3.226y 代码
时间: 2023-10-08 11:11:53 浏览: 81
以下是使用遗传算法计算并输出 f(x,y) 最大值的 Python 代码:
```python
import random
# 定义适应度函数
def fitness(x, y):
return (6.452 * pow((x + 0.1225 * y) * (cos(x) - cos(2 * y)), 2)) / (0.8 + pow(x - 4.2, 2) + 2 * pow(y - 7, 2)) + 3.226 * y
# 定义交叉函数
def crossover(parent1, parent2):
child1 = [parent1[0], parent2[1]]
child2 = [parent2[0], parent1[1]]
return child1, child2
# 定义变异函数
def mutation(child, mutation_rate):
if random.random() < mutation_rate:
if random.random() < 0.5:
child[0] += random.uniform(-0.1, 0.1)
else:
child[1] += random.uniform(-0.1, 0.1)
return child
# 初始化参数
population_size = 50
chromosome_length = 2
generation_limit = 1000
mutation_rate = 0.1
population = [[random.uniform(0, 10), random.uniform(0, 10)] for _ in range(population_size)]
generation = 0
# 开始进化
while generation < generation_limit:
# 计算适应度并排序
fitness_list = [fitness(chromosome[0], chromosome[1]) for chromosome in population]
population = [x for _, x in sorted(zip(fitness_list, population), key=lambda pair: pair[0], reverse=True)]
# 输出最优解
print("Generation:", generation, "Best solution:", population[0], "Fitness:", fitness_list[0])
# 选择两个父代进行交叉和变异
parent1 = population[random.randint(0, population_size - 1)]
parent2 = population[random.randint(0, population_size - 1)]
child1, child2 = crossover(parent1, parent2)
child1 = mutation(child1, mutation_rate)
child2 = mutation(child2, mutation_rate)
# 替换最差的两个个体
population[-1] = child1
population[-2] = child2
generation += 1
```
在上述代码中,我们首先定义了适应度函数 `fitness`,然后定义了交叉函数 `crossover` 和变异函数 `mutation`。接着初始化了遗传算法的参数,包括种群大小、染色体长度、进化代数等。然后进入主循环,每一轮循环中计算适应度并排序,输出最优解,然后选择两个父代进行交叉和变异,最后替换最差的两个个体。通过不断进化,遗传算法能够逐渐找到 f(x,y) 的最大值。
阅读全文
相关推荐






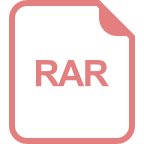










