中缀表达式转后缀表达式,后缀表达式计算的类定义·
时间: 2023-11-20 15:53:00 浏览: 85
中缀表达式转后缀表达式是一种常见的算法,它可以将中缀表达式转换为后缀表达式,使得计算更加方便。后缀表达式也叫做逆波兰表达式,它的计算方式是从左到右扫描表达式,遇到数字就压入栈中,遇到运算符就从栈中弹出两个数字进行计算,并将计算结果压入栈中,最终栈中只剩下一个数字,即为表达式的计算结果。
下面是一个C++的后缀表达式计算类的定义,其中包括了后缀表达式的计算方法和一些辅助方法:
```
#include <stack>
#include <string>
#include <sstream>
class PostfixCalculator {
public:
PostfixCalculator() {}
double calculate(std::string postfix) {
std::stack<double> s;
std::stringstream ss(postfix);
std::string token;
while (std::getline(ss, token, ' ')) {
if (isOperator(token)) {
double operand2 = s.top();
s.pop();
double operand1 = s.top();
s.pop();
double result = applyOperator(token, operand1, operand2);
s.push(result);
} else {
double operand = std::stod(token);
s.push(operand);
}
}
return s.top();
}
private:
bool isOperator(std::string token) {
return token == "+" || token == "-" || token == "*" || token == "/";
}
double applyOperator(std::string op, double operand1, double operand2) {
if (op == "+") {
return operand1 + operand2;
} else if (op == "-") {
return operand1 - operand2;
} else if (op == "*") {
return operand1 * operand2;
} else if (op == "/") {
return operand1 / operand2;
}
return 0;
}
};
```
使用该类可以方便地计算后缀表达式的值,例如:
```
PostfixCalculator calculator;
double result = calculator.calculate("1 2 3 - 4 * + 10 5 / +");
```
其中,后缀表达式为"1 2 3 - 4 * + 10 5 / +",计算结果为11.0。
阅读全文
相关推荐
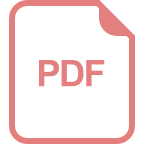
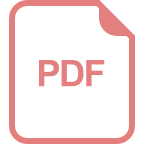
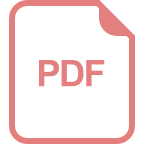















