java石头剪刀布游戏
时间: 2024-11-01 10:07:56 浏览: 9
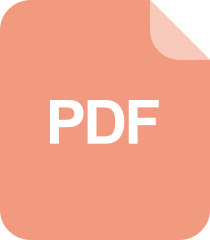
Java实现的剪刀石头布游戏示例
Java中的石头、剪刀、布游戏是一个常见的编程练习,用于展示基本的控制结构(如循环和条件判断)、用户输入处理以及随机数生成。游戏的基本流程通常是这样的:
1. 用户选择:提示玩家输入他们的选择(石头、剪刀或布),可以使用`Scanner`类获取用户的输入。
2. 程序选择:程序随机生成电脑的选择,通常通过`Math.random()`函数结合数组索引来实现。
3. 比较规则:根据规则判断胜负,例如石头胜剪刀,剪刀胜布,布胜石头。
4. 输出结果:显示比赛结果,告诉用户是赢了、输了还是平局。
5. 循环重复:为了增加游戏趣味性,可以设置让玩家再次玩或者退出游戏的选项。
这是一个简单的伪代码示例:
```java
import java.util.Scanner;
import java.util.Random;
public class RockPaperScissors {
public static void main(String[] args) {
Scanner input = new Scanner(System.in);
Random random = new Random();
String[] choices = {"石头", "剪刀", "布"};
while (true) {
System.out.println("请输入你的选择(石头、剪刀、布):");
String userChoice = input.nextLine().toLowerCase();
if (!choices.contains(userChoice)) {
System.out.println("无效的选择,请再试一次!");
continue;
}
int computerChoiceIndex = random.nextInt(choices.length);
String computerChoice = choices[computerChoiceIndex];
// 比较并输出结果
if (userChoice.equals(computerChoice)) {
System.out.println("平局!");
} else {
String result = determineWinner(userChoice, computerChoice);
System.out.println("你选的是" + userChoice + ",电脑选的是" + computerChoice + "," + result + "!");
}
System.out.print("再来一局吗?(y/n): ");
String playAgain = input.nextLine();
if (!playAgain.equalsIgnoreCase("y")) break;
}
}
private static String determineWinner(String userChoice, String computerChoice) {
if (userChoice.equals(computerChoice)) return "平局";
else if ((userChoice.equals("石头") && computerChoice.equals("剪刀")) ||
(userChoice.equals("剪刀") && computerChoice.equals("布")) ||
(userChoice.equals("布") && computerChoice.equals("石头"))) {
return "你赢了!";
} else {
return "电脑赢了!";
}
}
}
```
阅读全文
相关推荐
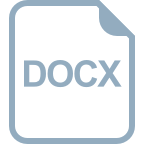
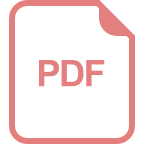
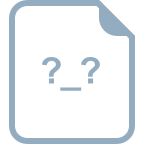
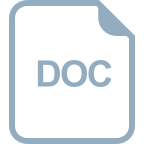
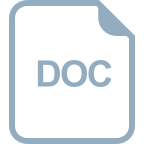
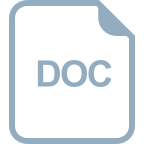
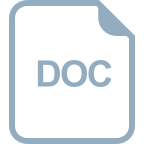









