(Sum integers) Write a program that passes an unspecified number of integers from command line and displays their total.
时间: 2024-12-20 09:16:03 浏览: 5
当需要编写一个程序来接收命令行输入的一系列整数,并计算它们的总和时,可以使用诸如Python这样的语言。下面是一个简单的Python示例,展示了如何实现这个功能:
```python
import sys
def sum_integers():
# 获取命令行参数
numbers = [int(num) for num in sys.argv[1:]]
# 计算总和
total = sum(numbers)
# 打印结果
print("给定整数的总和为:", total)
if __name__ == "__main__":
sum_integers()
```
在这个程序中:
1. `sys.argv` 是包含所有命令行参数的列表,我们从索引1开始取,因为索引0通常代表程序名。
2. 使用列表推导式将字符串类型的数字转换成整数。
3. `sum()` 函数计算列表中所有元素的总和。
4. 最后,打印出总和。
运行此程序时,在命令行输入如 "python script.py 1 2 3 4",它会显示 "给定整数的总和为: 10"。
相关问题
By java Write a program that passes an unspecified number of integers from command line and displays their total.
你可以通过创建一个简单的Java程序来实现这个功能。首先,我们需要从命令行获取用户输入的整数,然后计算它们的总和。下面是一个基本示例:
```java
import java.util.Scanner;
public class SumIntegers {
public static void main(String[] args) {
if (args.length == 0) {
System.out.println("Please provide at least one integer as a command-line argument.");
return;
}
int sum = 0;
for (int i = 0; i < args.length; i++) {
try {
// 将字符串转换为整数并累加到sum上
sum += Integer.parseInt(args[i]);
} catch (NumberFormatException e) {
System.out.println("Invalid input! Please enter only integers.");
return;
}
}
System.out.printf("The total sum of the numbers is: %d\n", sum);
}
}
```
在这个程序中,我们首先检查命令行参数的数量是否至少有一个。接着,遍历这些参数,尝试将每个参数解析为整数。如果无法转换成整数(例如,参数包含非数字字符),就捕获`NumberFormatException`并提示错误。最后,显示所有整数的总和。
运行这个程序时,你需要在命令行中提供至少一个整数作为参数,比如 `java SumIntegers 1 2 3 4 5`。
By java in English Write a program that passes an unspecified number of integers from command line and displays their total.
In Java, you can write a program to achieve this by using the `Scanner` class to read input from the command line and a variable to store the sum of the integers. Here's a simple example:
```java
public class SumIntegers {
public static void main(String[] args) {
// Create a Scanner object for command-line arguments
Scanner scanner = new Scanner(System.in);
// Initialize sum to zero
int sum = 0;
// Prompt user for the number of integers
System.out.println("Enter the number of integers followed by space-separated values:");
int count = scanner.nextInt();
// Iterate and add integers to the sum
for (int i = 0; i < count; i++) {
sum += scanner.nextInt();
}
// Display the total
System.out.printf("The total of %d integers is: %d%n", count, sum);
// Close the scanner
scanner.close();
}
}
```
To run this program, save it as "SumIntegers.java" and then compile and execute it from the command line, providing the desired numbers after the program name, like this:
```
javac SumIntegers.java
java SumIntegers 5 4 6 3 2
```
After running, it will display the total sum.
阅读全文
相关推荐
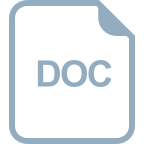
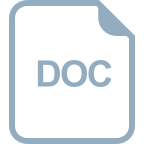
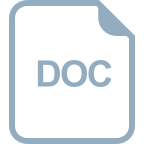













