请你用c++写一个prim算法
时间: 2024-12-24 17:19:42 浏览: 3
Prim's算法是一种用于寻找有向图中最短路径的贪心算法,通常用于求解最小生成树问题。以下是使用C++实现的一个简单版本:
```cpp
#include <iostream>
#include <vector>
#include <limits.h>
using namespace std;
// 定义边的数据结构
struct Edge {
int src, dest, weight;
};
// 边优先队列,使用pair作为比较依据
class PriorityQueue {
private:
vector<pair<int, int>> pq;
public:
void push(int weight, int node) {
pq.push_back({weight, node});
}
pair<int, int> top() {
return pq.front();
}
bool empty() {
return pq.empty();
}
void pop() {
pq.pop_front();
}
};
int primMST(vector<Edge>& edges, int numVertices) {
// 初始化距离数组和标记数组
vector<int> dist(numVertices, INT_MAX);
vector<bool> visited(numVertices, false);
// 将起始顶点的距离设为0
dist[0] = 0;
// 边优先队列
PriorityQueue priorityQueue;
for (Edge edge : edges) {
if (edge.weight < dist[edge.dest]) {
priorityQueue.push(edge.weight, edge.src);
}
}
priorityQueue.push(INT_MAX, -1); // 添加负无穷大以保证结束循环
while (!priorityQueue.empty()) {
int u = priorityQueue.top().second;
priorityQueue.pop();
// 如果u已经被访问过了,跳过
if (visited[u])
continue;
visited[u] = true;
// 更新邻接节点的距离
for (Edge edge : edges) {
if (!visited[edge.dest] && edge.src == u && edge.weight < dist[edge.dest]) {
dist[edge.dest] = edge.weight;
priorityQueue.push(dist[edge.dest], edge.dest);
}
}
}
// 最小生成树的总权重
int mstTotalWeight = 0;
for (int i = 1; i < numVertices; i++) {
mstTotalWeight += dist[i];
}
return mstTotalWeight;
}
int main() {
vector<Edge> edges = {{0, 1, 4}, {0, 7, 8}, {1, 2, 8}, {1, 6, 5},
{2, 3, 7}, {3, 4, 9}, {4, 5, 11}, {5, 6, 2},
{6, 7, 1}, {7, 8, 4}, {8, 9, 14}, {9, 10, 10}};
int numVertices = 10;
cout << "Minimum spanning tree total weight: " << primMST(edges, numVertices) << endl;
return 0;
}
```
这个程序首先初始化了距离数组和标记数组,然后将起始顶点的最短距离设为0,并将其加入优先队列。在while循环中,不断选择当前未访问且距离最近的顶点,并更新其相邻顶点的距离。最后,返回最小生成树的总重量。
阅读全文
相关推荐
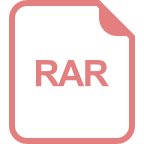
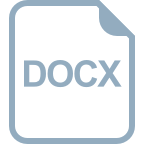

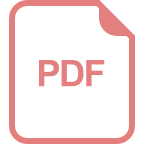
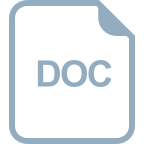
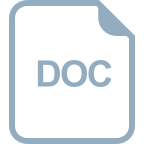
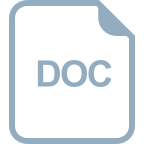









