帮我写C++代码实现prim算法
时间: 2024-08-15 08:02:00 浏览: 53
Prim's算法是一种用于求解图中最短路径树或最小生成树的贪心算法。以下是C++代码实现,这里假设图是以邻接矩阵表示:
```cpp
#include <vector>
#include <limits>
struct Edge {
int src, dest, weight;
};
bool compare(const Edge& e1, const Edge& e2) {
return e1.weight < e2.weight; // 按照权重升序比较
}
std::vector<int> primMST(int graph[][V], int V, int E) {
std::vector<int> mst(V, INT_MAX); // 初始化最短路径数组
std::priority_queue<Edge, std::vector<Edge>, decltype(compare)> pq(compare);
// 将起始节点(通常是0)加入优先队列,并设置其最短距离为0
mst = 0;
pq.push({0, 0, 0});
while (!pq.empty()) {
Edge current_edge = pq.top();
pq.pop();
if (mst[current_edge.src] != INT_MAX && mst[current_edge.dest] > mst[current_edge.src] + current_edge.weight) {
mst[current_edge.dest] = mst[current_edge.src] + current_edge.weight;
pq.push({current_edge.dest, current_edge.src, mst[current_edge.dest]});
}
}
return mst; // 返回最小生成树的边权值
}
int main() {
int graph[V][V];
// 填充你的邻接矩阵...
int V = sizeof(graph) / sizeof(graph);
int E = ...; // 图的边数
std::vector<int> result = primMST(graph, V, E);
// 打印结果...
return 0;
}
```
在这个代码中,`primMST`函数接收邻接矩阵、顶点数以及边的数量,然后通过Prim算法找到最小生成树。`compare`函数用于排序优先队列中的边。
阅读全文
相关推荐
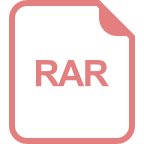
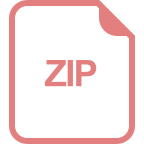
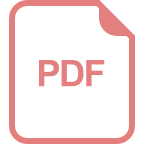
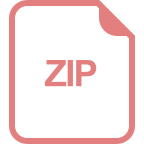
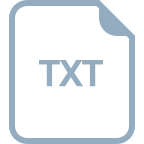
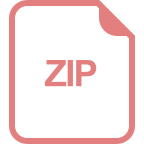
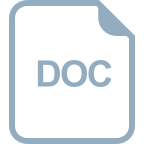
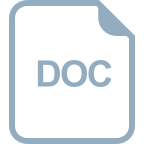
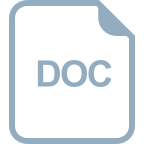
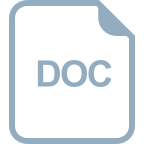
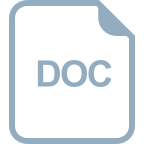
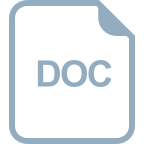
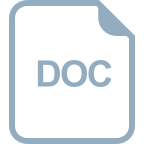




