#include "stdio.h" #include "unistd.h" #include "sys/types.h" #include "sys/stat.h" #include "fcntl.h" #include "stdlib.h" #include "string.h" #define HIGH 1 #define LOW 0 /* * @description : main 主程序 * @param - argc : argv 数组元素个数 * @param - argv : 具体参数 * @return : 0 成功;其他 失败 */ int main(int argc,char *argv[]) //argv[1]:表示要打开或关闭的设备文件名,例如 "/dev/led"; //argv[2]:表示要对设备进行的操作,取值为 0 或 1,分别表示关闭或打开设备。 { //printf("APP Run! \r\n"); int fd; int retvalue; char *filename; unsigned char databuf[1]; printf("APP Run! \r\n"); if(argc !=3)//两个参数argv[1],argv[2],3个参数(脚本文件名也算一个参数) { printf("Error Usage!!\r\n"); return -1; } filename=argv[1]; /* 打开 led 驱动 */ fd=open(filename,O_RDWR); if (fd<0) { printf("file %s open failed!\r\n",argv[1]); return -1; } databuf[0]=atoi(argv[2]); /* 要执行的操作:打开或关闭 */ /* 向设备驱动写数据 */ /* 向/dev/led 文件写入数据 */ retvalue=write(fd,&databuf,sizeof(databuf)); //printf("write date = %d \r\n",databuf); if(retvalue<0) { printf("LED Control Failed!\r\n"); close(fd); return -1; } /* 关闭设备 */ retvalue=close(fd); if(retvalue<0) { printf("file %s close failed!\r\n",argv[1]); return -1; } return 0; }为啥打印消息是这样的[ 46.882780] kernel received date = 1 APP Run! [ 46.887095] register date = 1c208a0:4
时间: 2024-03-29 17:39:45 浏览: 44
这是因为程序打开了一个设备文件,并向其中写入了数据。这个设备文件可能是一个驱动程序,驱动程序会将数据传递给内核,内核会打印出收到的数据。程序在打印出"APP Run!"之后,调用了write函数向设备文件中写入数据,随后内核打印出了收到的数据。最后程序关闭了设备文件。而[ 46.887095] register date = 1c208a0:4 这条消息可能是内核中某个模块的日志输出。
相关问题
#include "stdio.h" #include "unistd.h" #include "sys/types.h" #include "sys/stat.h" #include "fcntl.h" #include "stdlib.h" #include "string.h"有动态库不可以在ld-linux.so.3使用吗
是的,如果在程序中使用了某些依赖于 ld-linux-armhf.so.3 或 ld-linux-armel.so.3 动态链接器的共享库文件,而将程序链接到 ld-linux.so.3 上,则可能会出现运行时错误,导致程序无法正常运行。
这是因为不同的动态链接器实现之间可能存在差异,例如链接方式、搜索路径、符号解析等方面的差异,这些差异可能会影响共享库文件的加载和链接。因此,建议在选择动态链接器时,始终选择与目标系统架构相匹配的动态链接器,以确保程序能够正确地运行。
在你提供的代码中,使用了一些标准库函数,这些函数通常都是链接到 glibc 库中的。如果在程序中使用了其他共享库文件,需要根据具体情况进行选择合适的动态链接器,以确保程序能够正常运行。
#include <unistd.h> #include <string.h> #include <stdio.h> #include <sys/stat.h> #include <fcntl.h>
`#include <unistd.h>`是一个C语言的头文件,它包含了一些与系统调用相关的函数和常量。其中一些常用的函数包括`fork()`、`exec()`和`pipe()`等。`<string.h>`是另一个C语言的头文件,它包含了一些与字符串操作相关的函数,例如`strcpy()`和`strlen()`等。`<stdio.h>`是标准输入输出的头文件,它包含了一些与输入输出相关的函数,例如`printf()`和`scanf()`等。`<sys/stat.h>`是一个系统状态的头文件,它包含了一些与文件状态相关的函数和常量,例如`stat()`和`chmod()`等。`<fcntl.h>`是文件控制的头文件,它包含了一些与文件操作相关的函数和常量,例如`open()`和`close()`等。
以下是一个简单的示例代码,演示了如何使用这些头文件中的函数:
```c
#include <unistd.h>
#include <string.h>
#include <stdio.h>
#include <sys/stat.h>
#include <fcntl.h>
int main() {
// 使用unistd.h中的函数
int pid = fork();
if (pid == 0) {
// 子进程
printf("This is child process.\n");
} else if (pid > 0) {
// 父进程
printf("This is parent process.\n");
} else {
// 出错
perror("fork");
return 1;
}
// 使用string.h中的函数
char str1[10] = "Hello";
char str2[10];
strcpy(str2, str1);
printf("Copied string: %s\n", str2);
// 使用stdio.h中的函数
int num;
printf("Enter a number: ");
scanf("%d", &num);
printf("You entered: %d\n", num);
// 使用sys/stat.h和fcntl.h中的函数
int fd = open("file.txt", O_RDONLY);
struct stat fileStat;
fstat(fd, &fileStat);
printf("File size: %ld bytes\n", fileStat.st_size);
close(fd);
return 0;
}
```
这段代码演示了如何使用`fork()`函数创建子进程,使用`strcpy()`函数复制字符串,使用`scanf()`函数读取用户输入的数字,以及使用`open()`函数打开文件并使用`fstat()`函数获取文件大小。
阅读全文
相关推荐
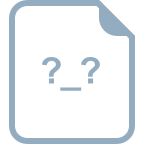
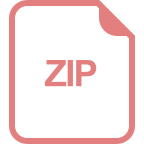
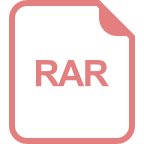














