将polynomial类用字典表示
时间: 2023-09-08 15:13:21 浏览: 44
可以将一个多项式表示为字典,其中字典的键是指数,字典的值是对应的系数。例如,多项式3x^2 + 2x + 1可以表示为字典{2:3, 1:2, 0:1}。以下是一个示例代码:
```python
class Polynomial:
def __init__(self, coeffs):
self.coeffs = coeffs
def __repr__(self):
return "Polynomial({})".format(self.coeffs)
def __add__(self, other):
new_coeffs = {}
for exp in set(self.coeffs.keys()) | set(other.coeffs.keys()):
new_coeffs[exp] = self.coeffs.get(exp, 0) + other.coeffs.get(exp, 0)
return Polynomial(new_coeffs)
def __mul__(self, other):
new_coeffs = {}
for exp1, coeff1 in self.coeffs.items():
for exp2, coeff2 in other.coeffs.items():
new_exp = exp1 + exp2
new_coeff = coeff1 * coeff2
if new_exp in new_coeffs:
new_coeffs[new_exp] += new_coeff
else:
new_coeffs[new_exp] = new_coeff
return Polynomial(new_coeffs)
```
使用这个类,我们可以用以下方式创建和操作多项式:
```python
p1 = Polynomial({2: 3, 1: 2, 0: 1})
p2 = Polynomial({3: 1, 1: 4, 0: 1})
print(p1) # 输出:Polynomial({2: 3, 1: 2, 0: 1})
print(p2) # 输出:Polynomial({3: 1, 1: 4, 0: 1})
p3 = p1 + p2
print(p3) # 输出:Polynomial({3: 1, 2: 3, 1: 6, 0: 2})
p4 = p1 * p2
print(p4) # 输出:Polynomial({5: 3, 4: 12, 3: 7, 2: 10, 1: 2, 0: 1})
```
相关推荐
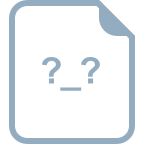
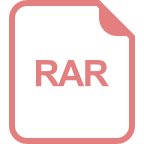
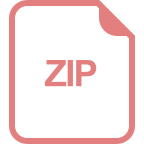














