用字典实现Polynomial
时间: 2024-04-29 13:21:41 浏览: 61
可以使用 Python 中的字典来实现多项式。我们可以把每个多项式的指数作为字典的键,系数作为键对应的值。例如,多项式 `3x^2 + 5x + 2` 可以表示为 `{2: 3, 1: 5, 0: 2}`。
下面是一个实现 Polynomial 类的示例代码:
```python
class Polynomial:
def __init__(self, coeffs):
self.coeffs = coeffs
def __str__(self):
terms = []
for exp, coeff in sorted(self.coeffs.items(), reverse=True):
if coeff == 0:
continue
elif exp == 0:
terms.append(str(coeff))
elif coeff == 1:
terms.append(f'x^{exp}')
else:
terms.append(f'{coeff}x^{exp}')
return ' + '.join(terms)
def __add__(self, other):
res = {}
for exp, coeff in self.coeffs.items():
res[exp] = coeff
for exp, coeff in other.coeffs.items():
res[exp] = res.get(exp, 0) + coeff
return Polynomial(res)
def __sub__(self, other):
res = {}
for exp, coeff in self.coeffs.items():
res[exp] = coeff
for exp, coeff in other.coeffs.items():
res[exp] = res.get(exp, 0) - coeff
return Polynomial(res)
def __mul__(self, other):
res = {}
for exp1, coeff1 in self.coeffs.items():
for exp2, coeff2 in other.coeffs.items():
exp = exp1 + exp2
coeff = coeff1 * coeff2
res[exp] = res.get(exp, 0) + coeff
return Polynomial(res)
```
在这个类中,`__init__` 方法接收一个字典作为参数,表示多项式的系数。`__str__` 方法将多项式转换为字符串表示形式,例如 `3x^2 + 5x + 2`。`__add__` 和 `__sub__` 方法分别实现了多项式的加法和减法。`__mul__` 方法实现了多项式的乘法。这些方法都返回一个新的 Polynomial 对象,以便支持链式调用。
这里是一个使用示例:
```python
p1 = Polynomial({2: 3, 1: 5, 0: 2})
p2 = Polynomial({1: 2, 0: 1})
print(p1) # 输出 "3x^2 + 5x + 2"
print(p2) # 输出 "2x + 1"
print(p1 + p2) # 输出 "3x^2 + 7x + 3"
print(p1 - p2) # 输出 "3x^2 + 3x + 1"
print(p1 * p2) # 输出 "6x^3 + 11x^2 + 12x + 2"
```
阅读全文
相关推荐
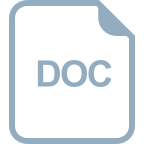
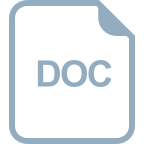
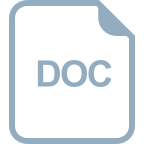


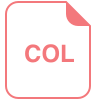







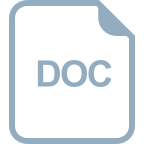
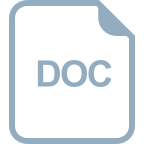
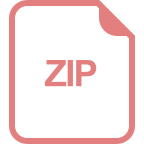
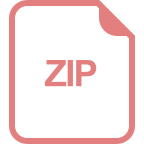