polynomial类
时间: 2023-12-30 14:52:12 浏览: 59
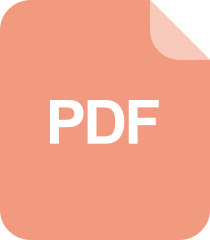
聚类是一个将数据集划分为若干组或簇的过程.pdf
Polynomial类是一个表示多项式的数据类型,可以用于多项式的存储、计算和操作。在该类中,通常使用一个数组来表示多项式的系数,每个元素对应于某个幂次项的系数。
下面是一个简单的Polynomial类的示例:
```java
public class Polynomial {
private double[] coefficients;
public Polynomial(double... coefficients) {
this.coefficients = coefficients;
}
public int degree() {
return coefficients.length - 1;
}
public double getCoefficient(int degree) {
return coefficients[degree];
}
public double evaluate(double x) {
double result = 0;
for (int i = coefficients.length - 1; i >= 0; i--) {
result = result * x + coefficients[i];
}
return result;
}
public Polynomial add(Polynomial other) {
int degree = Math.max(this.degree(), other.degree());
double[] result = new double[degree + 1];
for (int i = 0; i <= degree; i++) {
result[i] = this.getCoefficient(i) + other.getCoefficient(i);
}
return new Polynomial(result);
}
public Polynomial subtract(Polynomial other) {
int degree = Math.max(this.degree(), other.degree());
double[] result = new double[degree + 1];
for (int i = 0; i <= degree; i++) {
result[i] = this.getCoefficient(i) - other.getCoefficient(i);
}
return new Polynomial(result);
}
public Polynomial multiply(Polynomial other) {
double[] result = new double[this.degree() + other.degree() + 1];
for (int i = 0; i <= this.degree(); i++) {
for (int j = 0; j <= other.degree(); j++) {
result[i + j] += this.getCoefficient(i) * other.getCoefficient(j);
}
}
return new Polynomial(result);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
for (int i = this.degree(); i >= 0; i--) {
double coefficient = this.getCoefficient(i);
if (coefficient != 0) {
if (coefficient > 0 && sb.length() > 0) {
sb.append("+");
}
if (coefficient != 1 || i == 0) {
sb.append(coefficient);
}
if (i > 0) {
sb.append("x");
if (i > 1) {
sb.append("^").append(i);
}
}
}
}
return sb.toString();
}
}
```
在上述代码中,Polynomial类包含了以下操作:
- 构造函数:使用一个可变长度参数来表示多项式的系数。
- degree()方法:返回多项式的最高次项次数。
- getCoefficient(int degree)方法:返回多项式某个幂次项的系数。
- evaluate(double x)方法:计算多项式在某个值x处的值。
- add(Polynomial other)方法:将当前多项式与另一个多项式other相加,返回一个新的多项式。
- subtract(Polynomial other)方法:将当前多项式减去另一个多项式other,返回一个新的多项式。
- multiply(Polynomial other)方法:将当前多项式与另一个多项式other相乘,返回一个新的多项式。
- toString()方法:返回多项式的字符串表示。
使用Polynomial类可以方便地进行多项式的表示和计算,例如:
```java
Polynomial p1 = new Polynomial(1, 2, 3); // 3x^2 + 2x + 1
Polynomial p2 = new Polynomial(-1, 0, 1); // x^2 - 1
Polynomial sum = p1.add(p2); // 4x^2 + 2x
Polynomial product = p1.multiply(p2); // -x^4 + x^2 + 2x - 3
System.out.println(sum); // "4x^2+2x"
System.out.println(product); // "-x^4+x^2+2.0x-3.0"
```
阅读全文
相关推荐
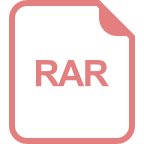






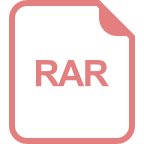
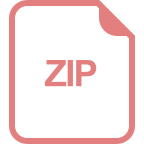


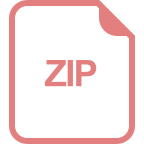
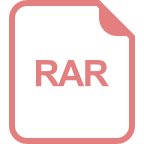
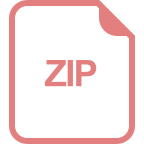

