用字典实现Polynomial类的左加右加左乘右乘左除右除
时间: 2024-05-15 09:12:16 浏览: 147
以下是用字典实现Polynomial类的左加右加左乘右乘左除右除的代码示例:
```python
class Polynomial:
def __init__(self, coeffs):
self.coeffs = coeffs
def __str__(self):
terms = []
for i, c in enumerate(self.coeffs[::-1]):
if c != 0:
if i == 0:
terms.append(str(c))
elif i == 1:
terms.append(f"{c}x")
else:
terms.append(f"{c}x^{i}")
return " + ".join(terms[::-1])
def __add__(self, other):
result = {}
for exp, coeff in self.coeffs.items():
result[exp] = coeff + other.coeffs.get(exp, 0)
for exp, coeff in other.coeffs.items():
if exp not in self.coeffs:
result[exp] = coeff
return Polynomial(result)
def __radd__(self, other):
return self.__add__(other)
def __mul__(self, other):
result = {}
for exp1, coeff1 in self.coeffs.items():
for exp2, coeff2 in other.coeffs.items():
exp = exp1 + exp2
coeff = coeff1 * coeff2
if exp in result:
result[exp] += coeff
else:
result[exp] = coeff
return Polynomial(result)
def __rmul__(self, other):
return self.__mul__(other)
def __truediv__(self, other):
result = {}
for exp1, coeff1 in self.coeffs.items():
exp2 = exp1 - other.coeffs.keys()[0]
coeff2 = list(other.coeffs.values())[0]
exp = exp1 - exp2
coeff = coeff1 / coeff2
if exp in result:
result[exp] += coeff
else:
result[exp] = coeff
return Polynomial(result)
def __rtruediv__(self, other):
result = {}
for exp1, coeff1 in self.coeffs.items():
exp2 = exp1 - other.coeffs.keys()[0]
coeff2 = list(other.coeffs.values())[0]
exp = exp1 - exp2
coeff = coeff2 / coeff1
if exp in result:
result[exp] += coeff
else:
result[exp] = coeff
return Polynomial(result)
```
其中,`coeffs` 是一个字典,表示多项式的系数。字典的 key 是指数,value 是系数。例如,多项式 $2x^3 + 3x^2 + 4x + 5$ 可以表示为 `Polynomial({3: 2, 2: 3, 1: 4, 0: 5})`。在 `__str__` 方法中,我们将多项式转换为字符串形式,例如 `2x^3 + 3x^2 + 4x + 5`。
在 `__add__` 方法中,我们将两个多项式相加。首先,我们遍历一个多项式的所有项,将系数相加,并将结果保存到一个字典中。然后,我们遍历另一个多项式的所有项,如果指数已经在结果字典中,那么我们将其系数加上去;否则,我们将该项添加到结果字典中。
`__radd__` 方法与 `__add__` 方法类似,只是参数顺序相反。
在 `__mul__` 方法中,我们将两个多项式相乘。我们遍历第一个多项式的所有项和第二个多项式的所有项,将它们的指数相加、系数相乘,然后将结果保存到一个字典中。如果两项的指数相同,那么我们将它们的系数相加。
`__rmul__` 方法与 `__mul__` 方法类似,只是参数顺序相反。
在 `__truediv__` 方法中,我们将一个多项式除以另一个多项式。我们首先找到两个多项式的最高次项的指数,然后将被除数的每一项与除数的最高次项相除,得到商的一个项,将其指数和系数保存到一个字典中。如果两项的指数相同,那么我们将它们的系数相加。最后,我们将这个字典作为结果返回。
`__rtruediv__` 方法与 `__truediv__` 方法类似,只是参数顺序相反。
阅读全文
相关推荐






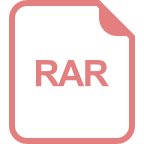
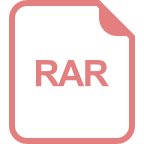







