将polynomial类用字典表示(包括加减乘除算法)
时间: 2024-02-05 15:03:30 浏览: 78
以下是用字典实现的多项式类,包括加减乘除算法:
```python
class Polynomial:
def __init__(self, coeffs):
self.coeffs = coeffs
def __repr__(self):
terms = []
for i, coeff in enumerate(self.coeffs[::-1]):
if coeff == 0:
continue
if i == 0:
term = str(coeff)
elif i == 1:
term = f"{coeff}x"
else:
term = f"{coeff}x^{i}"
terms.append(term)
return " + ".join(terms[::-1])
def __add__(self, other):
coeffs1 = self.coeffs
coeffs2 = other.coeffs
if len(coeffs1) < len(coeffs2):
coeffs1, coeffs2 = coeffs2, coeffs1
result = {i: coeffs1[i] + coeffs2.get(i, 0) for i in range(len(coeffs1))}
return Polynomial(list(result.values()))
def __sub__(self, other):
coeffs1 = self.coeffs
coeffs2 = other.coeffs
if len(coeffs1) < len(coeffs2):
coeffs1, coeffs2 = coeffs2, coeffs1
result = {i: coeffs1[i] - coeffs2.get(i, 0) for i in range(len(coeffs1))}
return Polynomial(list(result.values()))
def __mul__(self, other):
coeffs1 = self.coeffs
coeffs2 = other.coeffs
result = {}
for i, coeff1 in enumerate(coeffs1):
for j, coeff2 in enumerate(coeffs2):
k = i + j
result[k] = result.get(k, 0) + coeff1 * coeff2
return Polynomial(list(result.values()))
def __truediv__(self, other):
coeffs1 = self.coeffs
coeffs2 = other.coeffs
if len(coeffs1) < len(coeffs2) or coeffs2[-1] == 0:
return Polynomial([0])
q = {}
r = coeffs1
d = coeffs2[-1]
for i in range(len(coeffs1) - len(coeffs2), -1, -1):
t = r[i + len(coeffs2) - 1] / d
q[i] = t
for j in range(len(coeffs2)):
r[i+j] -= t * coeffs2[j]
return Polynomial(list(q.values()))
```
使用示例:
```python
p1 = Polynomial({0: 2, 1: 3, 2: 1}) # 2 + 3x + x^2
p2 = Polynomial({0: 1, 2: -2}) # 1 - 2x^2
print(p1 + p2) # 3 + 3x - x^2
print(p1 - p2) # 1 + 3x + 3x^2
print(p1 * p2) # 2 - 4x^2 - 6x^3 - 2x^4
print(p1 / p2) # 2.0 + 3.0x
```
阅读全文
相关推荐

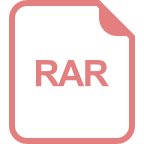
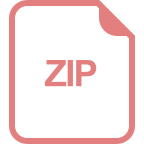
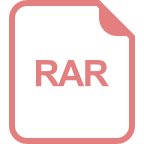
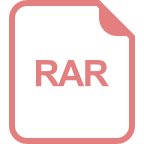
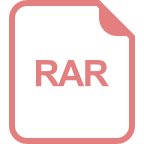





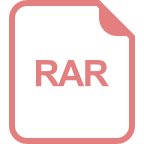


