开发类Polynomial(多项式)。在类内部,多项式由它的各个项组成的数组表示,每一项包含一个系数和一个指数。例如,项2x的系数为2,指数为4。开发一个完整的类,包含适当的构造函数、析构函数以及设置和获取函数。此外,Polynomial类还应提供下列重载运算符的能力: a)重载加法运算符(+),对两个Polynomial 做加法。 b)重载减法运算符(-),对两个Polynomial做减法。 c)重载赋值运算符,把一个Polynomal 赋给另一个。 d)重载乘法运算符(*),对两个Polynomial 做乘法。 e)重载加法赋值运算符(+=)、减法赋值运算符(-=)和乘法赋值运算符(*=)。
时间: 2024-02-25 17:59:09 浏览: 35
好的,我可以帮你解答这个问题。
以下是实现Polynomial类的代码示例:
```cpp
class Polynomial {
public:
// 构造函数
Polynomial(int n = 0) {
terms = new Term[n];
capacity = n;
length = 0;
}
// 拷贝构造函数
Polynomial(const Polynomial& other) {
capacity = other.capacity;
length = other.length;
terms = new Term[capacity];
for (int i = 0; i < length; i++) {
terms[i] = other.terms[i];
}
}
// 析构函数
~Polynomial() {
delete[] terms;
}
// 获取项数
int getLength() const {
return length;
}
// 获取某个项的系数
double getCoefficient(int exponent) const {
for (int i = 0; i < length; i++) {
if (terms[i].exponent == exponent) {
return terms[i].coefficient;
}
}
return 0;
}
// 设置某个项的系数
void setCoefficient(int exponent, double coefficient) {
for (int i = 0; i < length; i++) {
if (terms[i].exponent == exponent) {
terms[i].coefficient = coefficient;
return;
}
}
// 如果项不存在,则添加新项
if (length == capacity) {
// 扩容
capacity *= 2;
Term* newTerms = new Term[capacity];
for (int i = 0; i < length; i++) {
newTerms[i] = terms[i];
}
delete[] terms;
terms = newTerms;
}
terms[length++] = Term(exponent, coefficient);
}
// 重载加法运算符
Polynomial operator+(const Polynomial& other) const {
Polynomial result(length + other.length);
int i = 0, j = 0, k = 0;
while (i < length && j < other.length) {
if (terms[i].exponent > other.terms[j].exponent) {
result.terms[k++] = terms[i++];
} else if (terms[i].exponent < other.terms[j].exponent) {
result.terms[k++] = other.terms[j++];
} else {
double sum = terms[i++].coefficient + other.terms[j++].coefficient;
if (sum != 0) {
result.terms[k++] = Term(terms[i - 1].exponent, sum);
}
}
}
while (i < length) {
result.terms[k++] = terms[i++];
}
while (j < other.length) {
result.terms[k++] = other.terms[j++];
}
result.length = k;
return result;
}
// 重载减法运算符
Polynomial operator-(const Polynomial& other) const {
Polynomial result(length + other.length);
int i = 0, j = 0, k = 0;
while (i < length && j < other.length) {
if (terms[i].exponent > other.terms[j].exponent) {
result.terms[k++] = terms[i++];
} else if (terms[i].exponent < other.terms[j].exponent) {
result.terms[k++] = Term(other.terms[j].exponent, -other.terms[j].coefficient);
j++;
} else {
double diff = terms[i++].coefficient - other.terms[j++].coefficient;
if (diff != 0) {
result.terms[k++] = Term(terms[i - 1].exponent, diff);
}
}
}
while (i < length) {
result.terms[k++] = terms[i++];
}
while (j < other.length) {
result.terms[k++] = Term(other.terms[j].exponent, -other.terms[j].coefficient);
j++;
}
result.length = k;
return result;
}
// 重载赋值运算符
Polynomial& operator=(const Polynomial& other) {
if (this != &other) {
delete[] terms;
capacity = other.capacity;
length = other.length;
terms = new Term[capacity];
for (int i = 0; i < length; i++) {
terms[i] = other.terms[i];
}
}
return *this;
}
// 重载乘法运算符
Polynomial operator*(const Polynomial& other) const {
Polynomial result(length * other.length);
for (int i = 0; i < length; i++) {
for (int j = 0; j < other.length; j++) {
int exponent = terms[i].exponent + other.terms[j].exponent;
double coefficient = terms[i].coefficient * other.terms[j].coefficient;
result.setCoefficient(exponent, result.getCoefficient(exponent) + coefficient);
}
}
return result;
}
// 重载加法赋值运算符
Polynomial& operator+=(const Polynomial& other) {
*this = *this + other;
return *this;
}
// 重载减法赋值运算符
Polynomial& operator-=(const Polynomial& other) {
*this = *this - other;
return *this;
}
// 重载乘法赋值运算符
Polynomial& operator*=(const Polynomial& other) {
*this = *this * other;
return *this;
}
private:
struct Term {
int exponent;
double coefficient;
Term(int e = 0, double c = 0) : exponent(e), coefficient(c) {}
};
Term* terms;
int capacity;
int length;
};
```
在这个实现中,Polynomial类中的一个项由指数和系数两个成员变量组成,使用一个结构体Term表示。Polynomial类中使用一个Term数组来表示多项式,使用capacity、length来表示数组的容量和实际长度。实现了获取和设置某个项的系数的函数,以及各种运算符的重载函数。
在重载加法运算符和减法运算符时,需要注意两个多项式的指数可能不同,因此需要按照指数大小的顺序来合并多项式。在重载减法运算符时,需要将另一个多项式中的项的系数取相反数。
在重载乘法运算符时,使用两个for循环将两个多项式中的每一项相乘,并把结果加到新的多项式中。需要注意的是,两个多项式相乘后,可能会有指数相同的项,因此需要合并同类项,即将相同指数的项的系数相加。
在重载加法赋值运算符、减法赋值运算符和乘法赋值运算符时,分别调用对应的运算符重载函数,并将结果赋给当前对象。
相关推荐
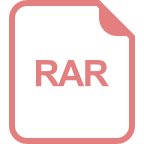
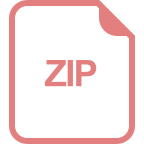














