By java in English Write a program that passes an unspecified number of integers from command line and displays their total.
时间: 2024-12-20 09:16:46 浏览: 2
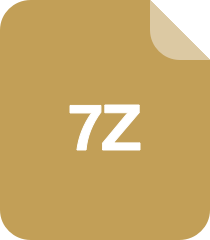
Linux Command Line for You and Me
In Java, you can write a program to achieve this by using the `Scanner` class to read input from the command line and a variable to store the sum of the integers. Here's a simple example:
```java
public class SumIntegers {
public static void main(String[] args) {
// Create a Scanner object for command-line arguments
Scanner scanner = new Scanner(System.in);
// Initialize sum to zero
int sum = 0;
// Prompt user for the number of integers
System.out.println("Enter the number of integers followed by space-separated values:");
int count = scanner.nextInt();
// Iterate and add integers to the sum
for (int i = 0; i < count; i++) {
sum += scanner.nextInt();
}
// Display the total
System.out.printf("The total of %d integers is: %d%n", count, sum);
// Close the scanner
scanner.close();
}
}
```
To run this program, save it as "SumIntegers.java" and then compile and execute it from the command line, providing the desired numbers after the program name, like this:
```
javac SumIntegers.java
java SumIntegers 5 4 6 3 2
```
After running, it will display the total sum.
阅读全文
相关推荐
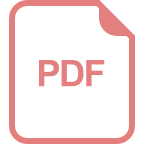
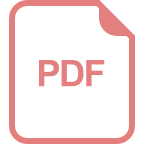















