c++实现有序数组的二分查找
时间: 2024-11-28 22:17:45 浏览: 54
在C++中,二分查找(Binary Search)是一种高效的查找算法,适用于已经排序的数组。它的工作原理是每次将数组分为两半,然后比较中间元素与目标值的关系,如果目标值小于中间元素,则在左半部分继续查找;如果大于,则在右半部分查找;直到找到目标值或确定目标值不存在于数组中。
以下是一个简单的C++函数实现二分查找:
```cpp
#include <iostream>
using namespace std;
int binarySearch(int arr[], int left, int right, int target) {
if (left > right) {
return -1; // 如果目标不在范围内,返回-1表示未找到
}
int mid = left + (right - left) / 2; // 计算中间索引
// 比较目标值与中间元素
if (arr[mid] == target) {
return mid; // 目标值找到,返回其位置
} else if (arr[mid] > target) {
return binarySearch(arr, left, mid - 1, target); // 在左半部分查找
} else {
return binarySearch(arr, mid + 1, right, target); // 在右半部分查找
}
}
int main() {
int arr[] = {1, 3, 5, 7, 9}; // 示例有序数组
int n = sizeof(arr) / sizeof(arr[0]);
int target = 5;
int result = binarySearch(arr, 0, n - 1, target);
if (result != -1) {
cout << "Element found at index " << result << endl;
} else {
cout << "Element not found in the array" << endl;
}
return 0;
}
```
阅读全文
相关推荐
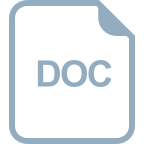
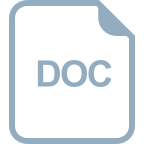
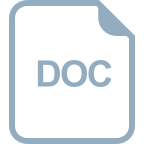
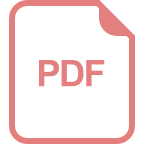
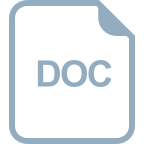


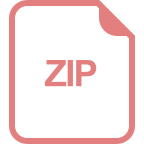
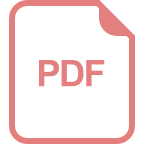
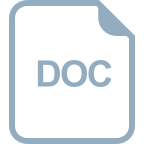
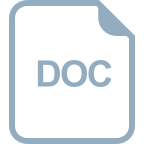
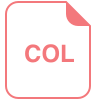






