electron 中使用 electron-dl 进行多个下载
时间: 2023-10-03 14:01:55 浏览: 74
在 Electron 中使用 electron-dl 进行多个下载的方法如下:
1. 首先,你需要定义一个包含多个下载项的数组。
2. 然后,使用 Promise.all() 方法来等待所有下载完成。在 Promise.all() 中,你可以使用 map() 方法来循环遍历下载项数组,并为每个下载项创建一个 Promise。
3. 在 Promise 的回调函数中,你可以使用 electron-dl 模块来进行文件下载。在下载完成后,你可以将下载项的状态设置为已完成。
以下是一个示例代码:
```javascript
const {app, BrowserWindow} = require('electron')
const {download} = require('electron-dl')
const path = require('path')
let mainWindow
function createWindow () {
mainWindow = new BrowserWindow({
width: 800,
height: 600,
webPreferences: {
nodeIntegration: true
}
})
mainWindow.loadFile('index.html')
mainWindow.on('closed', function () {
mainWindow = null
})
}
app.on('ready', createWindow)
app.on('window-all-closed', function () {
if (process.platform !== 'darwin') {
app.quit()
}
})
app.on('activate', function () {
if (mainWindow === null) {
createWindow()
}
})
const downloads = [
{
url: 'https://example.com/file1.txt',
properties: {directory: app.getPath('downloads')}
},
{
url: 'https://example.com/file2.txt',
properties: {directory: app.getPath('downloads')}
},
{
url: 'https://example.com/file3.txt',
properties: {directory: app.getPath('downloads')}
}
]
Promise.all(
downloads.map(downloadItem => {
return download(BrowserWindow.getFocusedWindow(), downloadItem.url, downloadItem.properties)
.then(dl => {
downloadItem.status = 'completed'
return dl.getSavePath()
})
.catch(error => {
downloadItem.status = 'failed'
console.error(error)
})
})
).then(results => {
console.log('All downloads complete:', results)
})
```
在上面的示例代码中,我们定义了一个包含三个下载项的数组 downloads。然后,我们使用 Promise.all() 方法来等待所有下载项完成。在 Promise.all() 中,我们使用 map() 方法来循环遍历下载项数组,并为每个下载项创建一个 Promise。在 Promise 的回调函数中,我们使用 electron-dl 模块来进行文件下载。在下载完成后,我们将下载项的状态设置为已完成。最后,我们使用 Promise.all() 的返回值来确定所有下载项是否已完成。
相关推荐
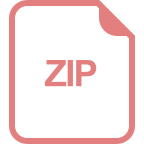
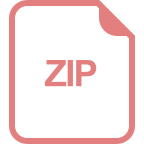
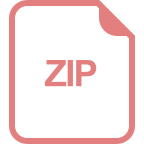















