python使用canny对‘img'文件夹中的灰度图像进行边缘检测,然后对检测后的图像进行霍夫变换,计算直线的角度,对灰度图像进行旋转,将旋转后的图像保存在新的文件夹中
时间: 2024-04-19 15:28:27 浏览: 87
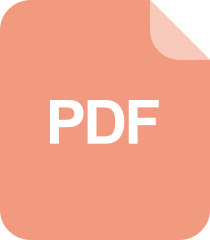
python实现canny边缘检测
下面是一个实现你所描述功能的示例代码:
```python
import cv2
import numpy as np
import os
def process_image(image_path, output_folder):
# 读取图像并转换为灰度图像
image = cv2.imread(image_path)
gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
# 边缘检测
edges = cv2.Canny(gray, 50, 150)
# 霍夫变换
lines = cv2.HoughLines(edges, 1, np.pi / 180, threshold=90)
# 计算直线角度的平均值
sum_angle = 0
count = 0
if lines is not None:
for line in lines:
rho, theta = line[0]
angle = theta * 180 / np.pi
sum_angle += angle
count += 1
avg_angle = sum_angle / count
# 对灰度图像进行旋转
rotated_image = rotate_image(gray, avg_angle)
# 保存旋转后的图像到新的文件夹中
filename = os.path.basename(image_path)
output_path = os.path.join(output_folder, filename)
cv2.imwrite(output_path, rotated_image)
def rotate_image(image, angle):
height, width = image.shape[:2]
center = (width // 2, height // 2)
# 构造旋转矩阵
rotation_matrix = cv2.getRotationMatrix2D(center, angle, 1.0)
# 进行旋转
rotated_image = cv2.warpAffine(image, rotation_matrix, (width, height))
return rotated_image
# 输入文件夹和输出文件夹的路径
input_folder = 'img'
output_folder = 'rotated_img'
# 创建输出文件夹
os.makedirs(output_folder, exist_ok=True)
# 处理每张图像
for filename in os.listdir(input_folder):
if filename.endswith(".jpg") or filename.endswith(".png"):
image_path = os.path.join(input_folder, filename)
process_image(image_path, output_folder)
```
在这个示例代码中,我们首先定义了 `process_image` 函数来处理单个图像。函数中,我们读取图像并将其转换为灰度图像,然后使用 `cv2.Canny` 函数进行边缘检测,接着使用 `cv2.HoughLines` 函数进行霍夫变换,计算直线角度的平均值。然后,我们使用自定义的 `rotate_image` 函数对灰度图像进行旋转,并将旋转后的图像保存到指定的输出文件夹中。
接下来,我们使用 `os.listdir` 函数遍历输入文件夹中的所有图像文件,对每个图像文件调用 `process_image` 函数进行处理。最后,我们将旋转后的图像保存到指定的输出文件夹中。
请确保将实际的输入文件夹路径和输出文件夹路径替换为正确的路径。
阅读全文
相关推荐
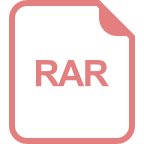
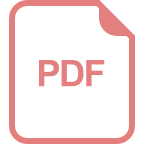
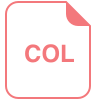
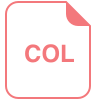












