while (isThreadRunning_) { std::unique_lock<std::mutex> lock(mutexQueue); while (processParaQueue.IsEmpty()) { if (!isThreadRunning_) { LCD_LOGGER_INFO("MonitorLCDAssistant Thread end"); return; } /* 此处会自动unlock锁,然后阻塞等待,直到cvQueue.notify_one来唤醒,被唤醒后会自动lock锁,然后wait函数才会返回。 wait返回后可以安全的使用mutex保护的临界区内的数据,此时mutex仍为上锁状态,故后面还需要手动释放锁。 */ cvQueue.wait(lock); cvQueue.wait(lock, [this] { return !processParaQueue.IsEmpty(); }); if (!isThreadRunning_) { lock.unlock(); return; } } bool isFindedProcess = processHelper.IsProcessRunning("LCDAssistant.exe"); if (!isFindedProcess) { cmdProcessParam = processParaQueue.Pop(); lock.unlock(); if (LoadUserProcessByServerPriviledge(cmdProcessName, cmdProcessParam) != RET_SUCCESS) { LCD_LOGGER_ERROR(L"LoadUserProcessByServerPriviledge error"); } } else { lock.unlock(); this_thread::sleep_for(chrono::milliseconds(msSleepTime)); } } bool IsEmpty() { std::lock_guard<std::mutex> lock(m_mutex); return m_queue.empty(); } 上面代码有没有问题
时间: 2024-02-14 17:29:09 浏览: 27
上面的代码有一些问题。
首先,在 `while (processParaQueue.IsEmpty())` 循环中,使用了两次连续的 `cvQueue.wait(lock)` 调用。这是不正确的。在第一次调用 `cvQueue.wait(lock)` 之后,线程会被阻塞,直到被其他线程通过 `cvQueue.notify_one()` 或 `cvQueue.notify_all()` 唤醒。然后,在被唤醒后执行第二次调用 `cvQueue.wait(lock, [this] { return !processParaQueue.IsEmpty(); })` 会再次阻塞线程,直到队列非空。因此,这样的连续调用是多余的,应该只保留一次调用即可。
另外,在 `if (!isThreadRunning_)` 分支中,使用了 `lock.unlock()` 来手动释放互斥锁。然而,在此之后的代码中,没有重新获取互斥锁来保护临界区的操作,这可能导致竞态条件的发生。正确的方式应该是在需要保护的代码段前后都使用互斥锁来加锁和解锁。
修正的代码如下:
```cpp
while (isThreadRunning_) {
std::unique_lock<std::mutex> lock(mutexQueue);
while (processParaQueue.IsEmpty()) {
if (!isThreadRunning_) {
LCD_LOGGER_INFO("MonitorLCDAssistant Thread end");
return;
}
cvQueue.wait(lock, [this] { return !processParaQueue.IsEmpty(); });
}
bool isFindedProcess = processHelper.IsProcessRunning("LCDAssistant.exe");
if (!isFindedProcess) {
cmdProcessParam = processParaQueue.Pop();
lock.unlock();
if (LoadUserProcessByServerPriviledge(cmdProcessName, cmdProcessParam) != RET_SUCCESS) {
LCD_LOGGER_ERROR(L"LoadUserProcessByServerPriviledge error");
}
} else {
this_thread::sleep_for(chrono::milliseconds(msSleepTime));
}
}
```
关于 `IsEmpty()` 函数的实现没有问题,使用了 `std::lock_guard<std::mutex>` 来保护临界区的访问,并返回队列是否为空的结果。
相关推荐
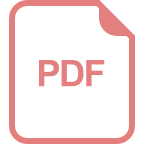
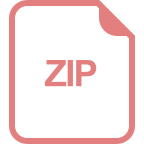















