图像的对极线校正的程序
时间: 2024-02-13 09:04:47 浏览: 116
这里给出一个简单的图像对极线校正的Python程序示例,使用OpenCV库进行实现:
```python
import cv2
import numpy as np
# 读取图像
img1 = cv2.imread('img1.jpg', 0)
img2 = cv2.imread('img2.jpg', 0)
# 提取SIFT特征点
sift = cv2.xfeatures2d.SIFT_create()
kp1, des1 = sift.detectAndCompute(img1, None)
kp2, des2 = sift.detectAndCompute(img2, None)
# 匹配特征点
bf = cv2.BFMatcher()
matches = bf.knnMatch(des1, des2, k=2)
# 筛选匹配点
good_matches = []
for m, n in matches:
if m.distance < 0.75 * n.distance:
good_matches.append(m)
# 计算本质矩阵
pts1 = np.float32([kp1[m.queryIdx].pt for m in good_matches]).reshape(-1, 1, 2)
pts2 = np.float32([kp2[m.trainIdx].pt for m in good_matches]).reshape(-1, 1, 2)
F, mask = cv2.findFundamentalMat(pts1, pts2, cv2.RANSAC, 3, 0.99)
# 计算对极线
lines1 = cv2.computeCorrespondEpilines(pts2, 2, F)
lines1 = lines1.reshape(-1, 3)
lines2 = cv2.computeCorrespondEpilines(pts1, 1, F)
lines2 = lines2.reshape(-1, 3)
# 对极线校正
img1_rectified = cv2.warpAffine(img1, np.eye(2), (img1.shape[1], img1.shape[0]))
img2_rectified = cv2.warpAffine(img2, np.eye(2), (img2.shape[1], img2.shape[0]))
for r, l in zip(pts1, lines1):
x0, y0 = map(int, [0, -l[2] / l[1]])
x1, y1 = map(int, [img1.shape[1], -(l[2] + l[0] * img1.shape[1]) / l[1]])
img1_rectified = cv2.line(img1_rectified, (x0, y0), (x1, y1), (0, 255, 0), 1)
for r, l in zip(pts2, lines2):
x0, y0 = map(int, [0, -l[2] / l[1]])
x1, y1 = map(int, [img2.shape[1], -(l[2] + l[0] * img2.shape[1]) / l[1]])
img2_rectified = cv2.line(img2_rectified, (x0, y0), (x1, y1), (0, 255, 0), 1)
# 显示结果
cv2.imshow('img1_rectified', img1_rectified)
cv2.imshow('img2_rectified', img2_rectified)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
以上程序中使用了SIFT特征点提取、双向暴力匹配、RANSAC算法估计本质矩阵、以及对极线校正等步骤。需要注意的是,该程序仅提供了基本的对极线校正功能,实际应用中可能需要进行更多的参数调整和优化,以提高校正的精度和效果。
阅读全文
相关推荐

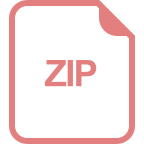
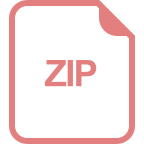
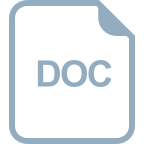
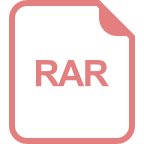
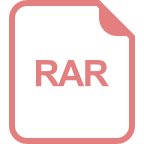
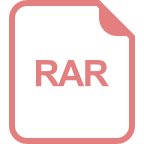
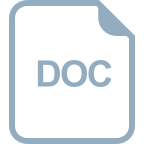
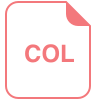
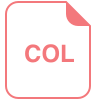

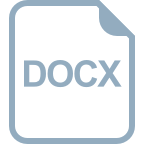