1.编写程序实现一元多项式的创建以及两个一元多项式相加和相乘的操作。
时间: 2024-11-12 10:32:42 浏览: 30
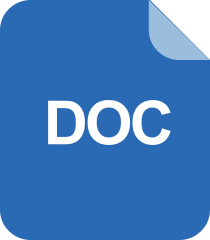
一元多项式的相乘(C链表实现).
在编程中,尤其是使用面向对象的语言,我们可以设计一个类来表示一元多项式。例如,在Python中,可以这样实现:
```python
class Polynomial:
def __init__(self, coefficients):
self.coefficients = coefficients
# 添加两个多项式
def add(self, other):
if len(self.coefficients) != len(other.coefficients):
raise ValueError("Polynomials must have the same degree to add.")
result = [a + b for a, b in zip(self.coefficients, other.coefficients)]
return Polynomial(result)
# 相乘两个多项式
def multiply(self, other):
if not isinstance(other, Polynomial):
raise TypeError("Multiplication is only supported between two polynomials.")
result = [0] * (len(self.coefficients) + len(other.coefficients) - 1)
for i, a in enumerate(self.coefficients):
for j, b in enumerate(other.coefficients):
result[i+j] += a * b
return Polynomial(result)
# 示例
p1 = Polynomial([2, 1, 0]) # x^2 + x
p2 = Polynomial([3, 0, 2]) # 3x + 2
sum_poly = p1.add(p2)
product_poly = p1.multiply(p2)
```
在这个例子中,`Polynomial` 类有系数列表作为属性,`add` 和 `multiply` 方法分别实现了多项式相加和相乘。注意这里假设输入的是同次数的一元多项式。
阅读全文
相关推荐
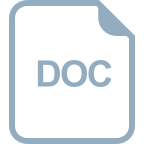
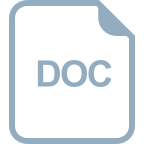





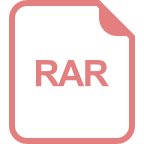
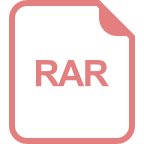
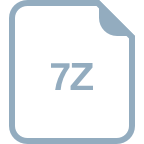







