用c语言编写程序实现一元多项式的创建以及两个一元多项式的相加和相乘的操作
时间: 2024-12-12 20:26:09 浏览: 16
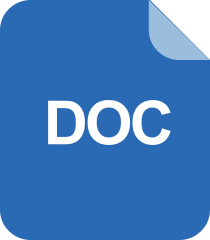
数据结构(C语言)用单链表存储一元多项式并实现两个多项式的相加运算.doc

在C语言中,我们可以使用结构体和指针来实现一元多项式。首先,定义一个结构体`Polynomial`表示一个多项式项,包括系数和指数:
```c
typedef struct {
double coefficient; // 系数
int exponent; // 指数
} Term;
typedef struct {
Term *terms;
int degree; // 最高次数
} Polynomial;
```
然后我们提供一些基本操作函数:
1. 创建多项式(初始化)
```c
Polynomial* create_polynomial(int n, double coefficients[], int exponents[]) {
Polynomial *poly = malloc(sizeof(Polynomial));
poly->degree = n - 1; // 减一,因为数组下标从0开始
poly->terms = malloc(n * sizeof(Term));
for (int i = 0; i < n; ++i) {
poly->terms[i].coefficient = coefficients[i];
poly->terms[i].exponent = exponents[i];
}
return poly;
}
```
2. 相加多项式
```c
void add_poly(Polynomial *a, Polynomial *b, Polynomial **result) {
if (!a || !b) return; // 检查输入是否有效
Polynomial *sum = malloc(sizeof(Polynomial));
sum->degree = max(a->degree, b->degree); // 取较大度数
sum->terms = malloc(sum->degree + 1 * sizeof(Term));
for (int i = 0; i <= sum->degree; ++i) {
double term_a = a->terms[i].coefficient;
double term_b = b->terms[i].coefficient;
sum->terms[i].coefficient = term_a + term_b;
}
*result = sum;
}
```
3. 相乘多项式(使用分配律)
```c
void multiply_poly(Polynomial *a, Polynomial *b, Polynomial **result) {
Polynomial *product = malloc(sizeof(Polynomial));
product->degree = a->degree + b->degree;
product->terms = malloc(product->degree + 1 * sizeof(Term));
memset(product->terms, 0, sizeof(Term)*(product->degree+1));
for (int i = 0; i <= a->degree; ++i) {
for (int j = 0; j <= b->degree; ++j) {
double term_a = a->terms[i].coefficient;
double term_b = b->terms[j].coefficient;
product->terms[i+j].coefficient += term_a * term_b;
}
}
*result = product;
}
```
阅读全文
相关推荐
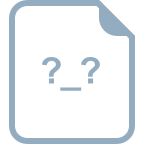
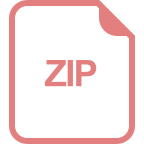







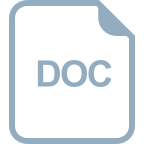







