编写程序实现一元多项式的创建以及两个一元多项式相加和相乘的操作。 C语言代码
时间: 2024-11-12 08:31:53 浏览: 83
在C语言中,我们可以使用结构体和函数来实现一元多项式的创建、相加和相乘操作。首先,定义一个多项式项的结构体:
```c
typedef struct {
double coefficient; // 系数
int exponent; // 指数
} PolynomialTerm;
```
接下来,定义一个多项式结构体,并包含一个数组来存储项:
```c
typedef struct {
int degree; // 阶数
PolynomialTerm terms[1]; // 动态数组,degree+1个元素
} Polynomial;
```
创建多项式的方法:
```c
void createPolynomial(Polynomial* poly, int degree, double coefficients[]) {
poly->degree = degree;
for (int i = 0; i <= degree; i++) {
poly->terms[i].coefficient = coefficients[i];
poly->terms[i].exponent = i;
}
}
```
相加两个多项式:
```c
void addPolynomials(Polynomial* result, const Polynomial* poly1, const Polynomial* poly2) {
if (poly1->degree > poly2->degree) {
Polynomial temp = *poly1;
*poly1 = *poly2;
*poly2 = temp;
}
for (int i = 0; i <= poly1->degree; i++) {
result->terms[i].coefficient += poly2->terms[i].coefficient;
result->degree = MAX(result->degree, i);
}
}
// 更新多项式的指数组成新的度数
#define UPDATE_DEGREE(terms, n) do { \
if (n > terms->degree) terms->degree = n; \
} while (0)
```
相乘两个多项式(需要维护系数和指数的累加):
```c
void multiplyPolynomials(Polynomial* result, const Polynomial* poly1, const Polynomial* poly2) {
Polynomial termResult;
memset(&termResult, 0, sizeof(termResult)); // 初始化
for (int i = 0; i <= poly1->degree; i++) {
for (int j = 0; j <= poly2->degree; j++) {
UPDATE_DEGREE(&result->terms, i + j);
result->terms[i + j].coefficient += poly1->terms[i].coefficient * poly2->terms[j].coefficient;
}
}
}
```
最后,为了方便操作,可以提供一些获取和打印多项式系数的辅助函数。
阅读全文
相关推荐
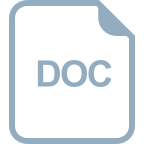
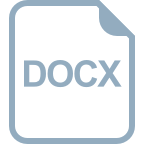
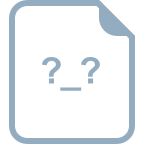






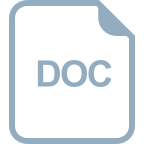
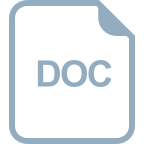
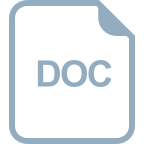






