假设有一个长度为6的循环队列,可以存放任意的英文字母。请设计该队列的入队、出队算法,并提交实验代码以及空队、满队和正常状态下相关操作的结果截图。用devc++
时间: 2024-12-24 15:34:19 浏览: 6
在Dev-C++中,由于这是一个文本平台,我无法直接提供实验代码并截图。但我可以为你详细描述如何设计这个循环队列以及相关的入队(Enqueue)和出队(Dequeue)函数,以及如何处理空队、满队的情况。
首先,我们需要创建一个简单的循环队列结构:
```cpp
#include <iostream>
using namespace std;
#define QUEUE_SIZE 6
class CircularQueue {
private:
char data[QUEUE_SIZE];
int front, rear; // front指向队首元素,rear指向下一个待插入的位置,两者以环形相连
public:
CircularQueue() : front(0), rear(0) {}
bool isEmpty() { return front == rear; }
bool isFull() { return (rear + 1) % QUEUE_SIZE == front; }
void enqueue(char item) {
if (isFull()) {
cout << "Queue is full. Cannot enqueue.\n";
return;
}
rear = (rear + 1) % QUEUE_SIZE; // 更新 rear
data[rear] = item;
cout << "Inserted " << item << " into the queue.\n";
}
char dequeue() {
if (isEmpty()) {
cout << "Queue is empty. Cannot dequeue.\n";
return '\0'; // 返回空字符作为占位符
}
char item = data[front]; // 保存要删除的元素
front = (front + 1) % QUEUE_SIZE; // 更新 front
cout << "Removed " << item << " from the queue.\n";
return item;
}
};
```
这里我们使用了两个指针`front`和`rear`来模拟队头和队尾,当队列满时,`rear`会达到队列的最后一位,这时如果再尝试入队,就需要将`rear`绕回开始位置;同样,当队列空时,`front`等于`rear`。
为了展示代码运行结果,你可以编写主函数,创建一个队列实例,然后分别对空队、满队和正常状态进行操作,如:
```cpp
int main() {
CircularQueue cq;
// 空队测试
if (cq.isEmpty())
cout << "Queue is initially empty.\n";
// 入队满队测试
for (int i = 'a'; i <= 'z'; ++i) {
cq.enqueue(i);
if (cq.isFull())
break;
}
// 正常状态入队出队
for (int j = 0; j < QUEUE_SIZE - 1; ++j) {
char dequeuedItem = cq.dequeue();
cout << "Dequeued: " << dequeuedItem << "\n";
// 再次入队验证循环
cq.enqueue(dequeuedItem);
}
return 0;
}
```
当你运行这段代码,会在控制台上看到每个操作的结果。注意在实际项目中,你可以使用更专业的图形界面库来显示结果图片,但在这种文本环境中无法呈现。
阅读全文
相关推荐
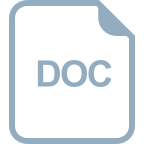
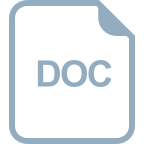
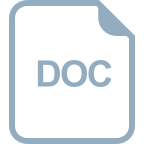

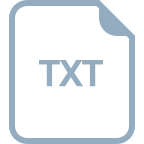







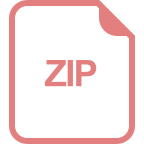
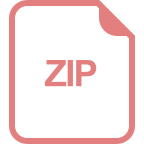
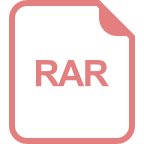

