Use a once for loop to print an isosceles triangle with “*”
时间: 2024-09-10 13:15:43 浏览: 84
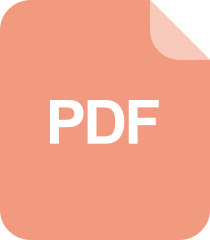
Isosceles正交注记 (2002年)
To create an isosceles triangle using a single `for` loop and asterisks (`*`), you can follow these steps:
1. First, determine the number of rows for the triangle based on user input or a fixed size.
2. Use nested loops within the main `for` loop to handle each row of the triangle.
3. For the first half of the rows (up to the middle), print spaces followed by asterisks, with the number of asterisks increasing by one in each step.
4. For the second half of the rows, print only asterisks, again with the number decreasing by one in each step.
Here's an example implementation in Python:
```python
# Assuming we know the desired height of the triangle
rows = 5
# Loop through each row
for i in range(1, rows + 1):
# Print spaces before the asterisks
print(' ' * (rows - i), end='')
# Handle the left and right halves of the triangle separately
if i <= rows // 2:
# Left half: increase asterisk count
asterisks = i
print('*' * asterisks)
else:
# Right half: decrease asterisk count
asterisks = rows - i + 1
print('*' * asterisks)
# Go to the next line after printing a row
print()
```
This code will produce an isosceles triangle with " *" characters, where the number of stars doubles for each row until it reaches the middle and then halves as it approaches the bottom.
阅读全文
相关推荐
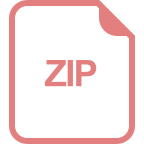
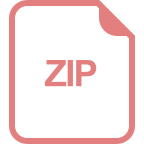
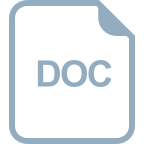














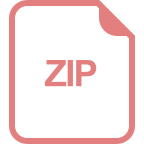