python cv code GaussianBlur
时间: 2024-12-23 15:10:59 浏览: 5
在Python中,使用OpenCV库进行高斯模糊处理可以使用以下代码:
```python
import cv2
import numpy as np
# 读取图像
img = cv2.imread('image.jpg')
# 对图像进行高斯模糊处理
blurred = cv2.GaussianBlur(img, (5, 5), 0)
# 显示原图和处理后的图像
cv2.imshow('Original Image', img)
cv2.imshow('Blurred Image', blurred)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
这段代码首先使用`cv2.imread`函数读取图像文件。然后,使用`cv2.GaussianBlur`函数对图像进行高斯模糊处理。该函数的第一个参数是要处理的图像,第二个参数是一个元组,指定了高斯核的大小,通常是奇数大小(例如(5,5)表示一个5x5的核)。第三个参数是标准差,它决定了高斯核的宽度。标准差越大,模糊效果越明显。在这个例子中,标准差被设置为0,这意味着高斯核的大小是固定的。
最后,使用`cv2.imshow`函数显示原始图像和处理后的图像。请注意,您需要安装OpenCV库才能运行此代码。如果您尚未安装OpenCV,可以使用pip安装:`pip install opencv-python`。
相关问题
opencv with cuda GaussianBlur on a video in python
To apply GaussianBlur on a video using OpenCV with CUDA in Python, you can use the following code:
```
import cv2
# Load the video
cap = cv2.VideoCapture('input_video.mp4')
# Create a CUDA enabled GaussianBlur object
blur = cv2.cuda.createGaussianFilter(cap.get(cv2.CAP_PROP_FRAME_HEIGHT), cap.get(cv2.CAP_PROP_FRAME_WIDTH), (3, 3), 0, cv2.CV_32F)
# Process each frame of the video
while True:
# Capture a frame
ret, frame = cap.read()
if not ret:
break
# Convert the frame to a CUDA enabled matrix
src = cv2.cuda_GpuMat(frame)
# Apply GaussianBlur on the frame
dst = blur.apply(src)
# Convert the CUDA enabled matrix to a numpy array
result = dst.download()
# Display the resulting frame
cv2.imshow('frame', result)
# Wait for key press
if cv2.waitKey(1) == ord('q'):
break
# Release the video and cleanup
cap.release()
cv2.destroyAllWindows()
```
In this code, we first load the video using `cv2.VideoCapture()`. We then create a CUDA enabled GaussianBlur object using `cv2.cuda.createGaussianFilter()`. We then process each frame of the video in a loop, where we first convert the frame to a CUDA enabled matrix using `cv2.cuda_GpuMat()`. We then apply GaussianBlur on the CUDA enabled matrix using `blur.apply()`. We then convert the CUDA enabled matrix to a numpy array using `dst.download()`. Finally, we display the resulting frame using `cv2.imshow()` and wait for a key press using `cv2.waitKey()`.
import cv2 import numpy as np def getContours(img): contours, hierarchy = cv2.findContours( img, cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_NONE ) for cnt in contours: area = cv2.contourArea(cnt) print(area) if area > 500: cv2.drawContours(imgContour, cnt, -1, (255, 0, 0), 3) path = "/home/robot/code.py/shape.jpg" img = cv2.imread(path) imgContour = img.copy() imgGray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY) imgBlur = cv2.GaussianBlur(imgGray, (5, 5), 1) imgCanny = cv2.Canny(imgBlur, 50, 50) getContours(imgCanny) imgBlank = np.zeros_like(img) cv2.imshow("Original", img) cv2.imshow("Gray", imgGray) cv2.imshow("Blur", imgBlur) cv2.imshow("Canny", imgCanny) cv2.imshow("Blank", imgContour) cv2.waitKey(0)有什么bug
代码中存在两个问题:
1. 在导入 cv2 模块时,应该使用以下方式:
```python
import cv2
import numpy as np
```
2. 在调用 ``cv2.drawContours()`` 函数时,需要将第一个参数改为 ``imgContour``,因为 ``imgContour`` 是我们要绘制轮廓的图像。
修改后的代码如下:
```python
import cv2
import numpy as np
def getContours(img):
contours, hierarchy = cv2.findContours(
img, cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_NONE
)
for cnt in contours:
area = cv2.contourArea(cnt)
print(area)
if area > 500:
cv2.drawContours(imgContour, cnt, -1, (255, 0, 0), 3)
path = "/home/robot/code.py/shape.jpg"
img = cv2.imread(path)
imgContour = img.copy()
imgGray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
imgBlur = cv2.GaussianBlur(imgGray, (5, 5), 1)
imgCanny = cv2.Canny(imgBlur, 50, 50)
getContours(imgCanny)
imgBlank = np.zeros_like(img)
cv2.imshow("Original", img)
cv2.imshow("Gray", imgGray)
cv2.imshow("Blur", imgBlur)
cv2.imshow("Canny", imgCanny)
cv2.imshow("Contour", imgContour)
cv2.waitKey(0)
```
阅读全文
相关推荐
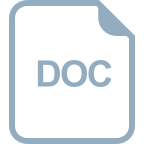
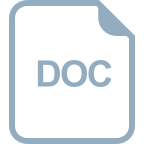
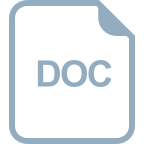
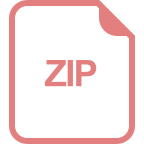
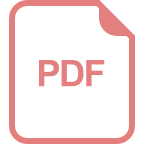
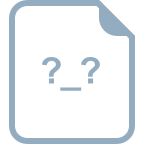
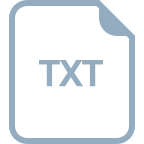
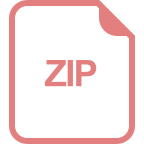
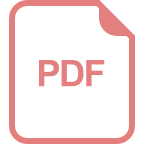
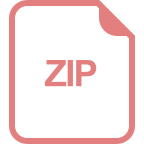
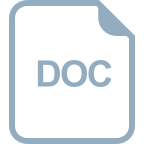
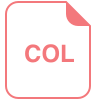
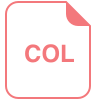
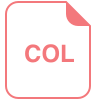
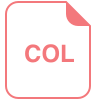
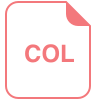
