输入年月日,格式2020-10-26,输出这一天是该年的第几天,第几周。检查日期,抛出异常。c++
时间: 2024-09-28 16:04:17 浏览: 46
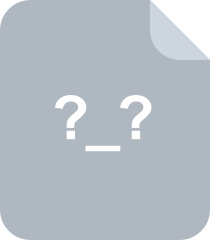
输入年月日计算第几天.md
在C++中,你可以通过`<ctime>`库来实现这个功能。首先,你需要包含头文件并创建一个函数来处理日期转换。这是一个简单的示例:
```cpp
#include <iostream>
#include <ctime>
#include <stdexcept>
int getDayOfWeekAndDayOfMonth(const std::string& dateStr) {
struct tm time_struct;
std::istringstream ss(dateStr);
ss >> std::get_time(&time_struct, "%Y-%m-%d");
// 检查日期是否有效
if (ss.fail() || !std::mktime(&time_struct)) {
throw std::invalid_argument("Invalid date format. Please use YYYY-MM-DD.");
}
// 获取当前时间的结构体,并减去给定日期,计算差值天数
auto now = std::chrono::system_clock::now();
auto now_tm = std::localtime(std::chrono::system_clock::to_time_t(now));
int days_since_epoch = static_cast<int>(std::chrono::duration_cast<std::chrono::days>(now - now_tm).count());
int day_of_year = time_struct.tm_yday; // 日历年中的第几天
int week_day = time_struct.tm_wday + 1; // 一周中的第一天是Sunday,所以加1
return {day_of_year, week_day};
}
int main() {
try {
std::string date_str = "2020-10-26";
auto [day_of_year, week_day] = getDayOfWeekAndDayOfMonth(date_str);
std::cout << "This date is the " << day_of_year << "th day of the year and it's on the " << week_day << "th week.\n";
} catch (const std::invalid_argument& e) {
std::cerr << e.what() << '\n';
}
return 0;
}
```
在这个例子中,我们首先尝试从给定的字符串解析日期,如果失败就抛出`std::invalid_argument`异常。然后,我们计算输入日期是一年中的第几天以及是一周中的哪一天。
阅读全文
相关推荐
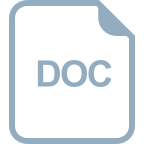
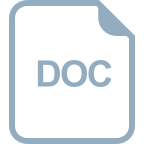
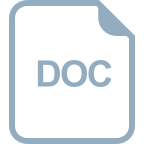














