Write+a+program+that+prints+the+next+character+for+the+corresponding+three+characters+given+to+the+p
时间: 2023-11-19 14:04:53 浏览: 88
以下是一个Python程序,它将打印给定字符串中每个字符的下一个字符:
```python
input_str = input("Enter a string: ")
for i in range(len(input_str)):
if i < len(input_str) - 2:
print(chr(ord(input_str[i]) + 1), end="")
elif i == len(input_str) - 2:
print(chr(ord(input_str[i]) + 1))
```
相关问题
Write+a+program++in+c+that+calculates+the+sum+of+the+digits+of+a+positive+integer+typed+in+from+the+keyboard.+For+example
Sure! Here's an example program in C that calculates the sum of the digits of a positive integer typed in from the keyboard:
```
#include <stdio.h>
int main() {
int num, sum = 0, digit;
printf("Enter a positive integer: ");
scanf("%d", &num);
while (num > 0) {
digit = num % 10;
sum += digit;
num /= 10;
}
printf("The sum of the digits is %d\n", sum);
return 0;
}
```
This program first prompts the user to enter a positive integer, then reads in the integer using `scanf()`. It then uses a `while` loop to repeatedly extract the rightmost digit of the integer using the modulus operator `%`, add it to a running sum, and remove that digit from the integer by dividing by 10. Once the entire integer has been processed, the program prints out the final sum.
I hope that helps! Let me know if you have any further questions.
Write a C program that inputs three strings that represent integers, converts the strings to integers sums the values, and prints the total of their values.
好的,以下是一个符合您要求的 C 语言程序:
```c
#include <stdio.h>
#include <stdlib.h> // 包含 atoi 函数
int main() {
// 定义需要输入的三个字符串
char str1[100], str2[100], str3[100];
// 输入三个字符串
printf("Please enter the first number: ");
scanf("%s", str1);
printf("Please enter the second number: ");
scanf("%s", str2);
printf("Please enter the third number: ");
scanf("%s", str3);
// 将三个字符串转为整数并求和
int num1 = atoi(str1);
int num2 = atoi(str2);
int num3 = atoi(str3);
int sum = num1 + num2 + num3;
// 输出结果
printf("The sum of the three numbers is: %d\n", sum);
return 0;
}
```
程序首先提示用户输入三个字符串,然后使用 `scanf` 函数读取用户的输入。接着,使用 `atoi` 函数将三个字符串转换为整数,并求它们的和。最后,输出结果。
阅读全文
相关推荐
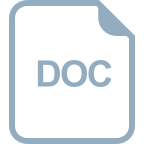














