实现顺序表类的定义、顺序表的插入、删除、显示、定位等基本操作:实现单链表类的定义、单链表的构造、插入、删除、定位、显示、销毁等基本操作
时间: 2024-10-12 22:15:20 浏览: 27
**顺序表类(arrList)的定义与基本操作**[^1]:
1. **定义**:
```cpp
template <class T> class arrList : public LinearList<T> {
// ...其他成员变量和方法...
};
```
2. **插入**: 在指定位置插入元素,如果位置超出范围会自动调整长度。
```cpp
void insert(int position, const T& value) {
if (position >= 0 && position <= maxSize - 1) {
alist[position] = value;
curLen++;
}
}
```
3. **删除**: 删除指定位置的元素,注意更新长度和可能的元素位移。
```cpp
void remove(int position) {
if (position >= 0 && position < curLen) {
memmove(&alist[position], &alist[position + 1], sizeof(T) * (curLen - position - 1));
alist[curLen - 1] = nullptr; // 或者置零,视情况而定
curLen--;
}
}
```
4. **显示**: 遍历并打印顺序表元素。
```cpp
void display() {
for (int i = 0; i < curLen; ++i) {
std::cout << alist[i] << " ";
}
std::cout << "\n";
}
5. **定位**: 获取某个元素的索引,如果不存在则返回无效位置。
```cpp
int find_position(const T& value) {
for (int i = 0; i < curLen; ++i) {
if (alist[i] == value) return i;
}
return -1; // 表示未找到
}
```
**单链表类的定义与基本操作**:
1. **定义**:
```cpp
class Node {
T data;
Node* next;
};
template <class T> class SingleLinkedList {
Node* head;
// ...其他成员变量和方法...
};
```
2. **构造**:
```cpp
SingleLinkedList() { head = nullptr; }
```
3. **插入**:
```cpp
void insert(const T& value) {
Node* newNode = new Node{value, nullptr};
if (!head) {
head = newNode;
} else {
Node* current = head;
while (current->next != nullptr) {
current = current->next;
}
current->next = newNode;
}
}
```
4. **删除**:
```cpp
void remove(const T& value) {
if (head) {
Node* current = head;
if (current->data == value) {
head = current->next;
delete current;
return;
}
while (current->next != nullptr && current->next->data != value) {
current = current->next;
}
if (current->next != nullptr) {
current->next = current->next->next;
delete current->next;
}
}
}
5. **定位**:
```cpp
Node* find(const T& value) {
Node* current = head;
while (current != nullptr) {
if (current->data == value) return current;
current = current->next;
}
return nullptr; // 表示未找到
}
6. **显示**:
```cpp
void display() {
Node* current = head;
while (current != nullptr) {
std::cout << current->data << " ";
current = current->next;
}
std::cout << "\n";
}
7. **销毁**:
```cpp
~SingleLinkedList() {
Node* temp = nullptr;
while (head != nullptr) {
temp = head;
head = head->next;
delete temp;
}
}
```
阅读全文
相关推荐
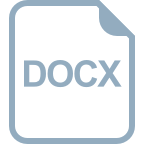
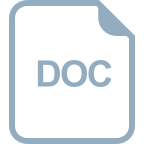
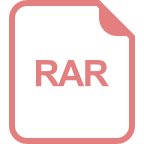
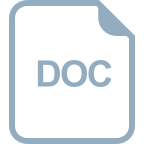
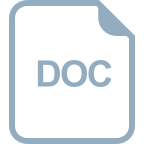
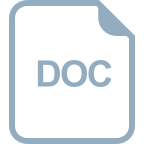
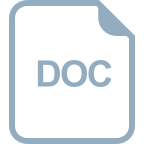
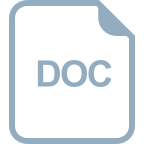
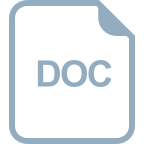
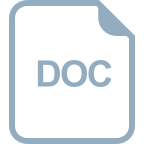
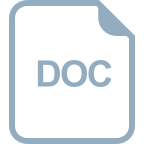
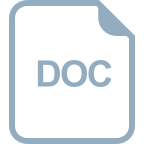
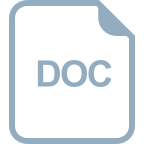
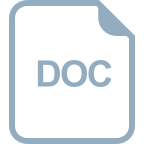
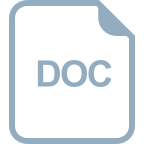
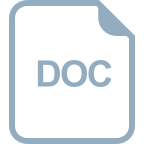


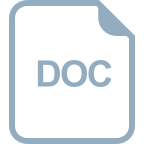