帮我写一个用jupyter爬取新华网首页内容的代码
时间: 2024-10-24 21:19:29 浏览: 30
在Jupyter Notebook中,我们可以使用Python的requests库来发送HTTP请求,并结合BeautifulSoup库解析HTML内容。以下是一个简单的例子,演示如何爬取新华网(http://www.xinhuanet.com/)首页的新闻标题:
```python
# 导入需要的库
import requests
from bs4 import BeautifulSoup
# 新华网首页URL
url = "http://www.xinhuanet.com/"
# 发送GET请求并获取页面内容
response = requests.get(url)
# 检查请求是否成功
if response.status_code == 200:
# 解析HTML内容
soup = BeautifulSoup(response.text, 'lxml')
# 找到包含新闻标题的区域,这里假设是class为"newslist"
titles = soup.find_all(class_='newslist') or soup.select('.newslist')
# 遍历提取每个标题
for title in titles:
headline = title.get_text() # 获取文本
print(headline)
else:
print(f"请求失败,状态码:{response.status_code}")
#
相关问题
用jupyter爬取微博热搜的代码
好的,以下是一个在jupyter中爬取微博热搜的代码:
```python
import requests
from bs4 import BeautifulSoup
url = 'https://s.weibo.com/top/summary?cate=realtimehot'
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
hot_list = soup.select('.list_a > tr')
for hot in hot_list:
rank = hot.select_one('.td-01.ranktop').text.strip()
title = hot.select_one('.td-02 > a').text.strip()
heat = hot.select_one('.td-02 > span').text.strip()
print(rank, title, heat)
```
将代码复制粘贴到jupyter中的代码单元格中,执行即可。
使用jupyter爬取晋江文学网
首先,你需要安装以下几个库:requests、beautifulsoup4 和 pandas。在Jupyter Notebook中打开一个新的Python文件,然后按照以下步骤开始爬取晋江文学网上的小说数据:
1. 导入所需的库:
```python
import requests
from bs4 import BeautifulSoup
import pandas as pd
```
2. 确定要爬取的小说的URL:
```python
url = 'https://www.jjwxc.net/onebook.php?novelid=123456'
```
请将"123456"替换为你想要爬取的小说的实际ID号。
3. 使用requests库获取HTML源代码:
```python
response = requests.get(url)
html = response.content
```
4. 使用BeautifulSoup库解析HTML源代码:
```python
soup = BeautifulSoup(html, 'html.parser')
```
5. 找到包含小说章节列表的HTML元素:
```python
chapter_list = soup.find('div', {'class': 'booklast'})
```
6. 找到所有小说章节的链接和标题:
```python
chapter_links = chapter_list.find_all('a')
chapter_titles = [chapter.text for chapter in chapter_links]
chapter_urls = ['https://www.jjwxc.net/' + chapter.get('href') for chapter in chapter_links]
```
7. 遍历所有章节链接,获取每章节的HTML源代码:
```python
chapter_html = []
for chapter_url in chapter_urls:
chapter_response = requests.get(chapter_url)
chapter_html.append(chapter_response.content)
```
8. 使用BeautifulSoup库解析每章节的HTML源代码,并提取章节内容:
```python
chapter_content = []
for chapter in chapter_html:
chapter_soup = BeautifulSoup(chapter, 'html.parser')
chapter_content.append(chapter_soup.find('div', {'class': 'noveltext'}).text)
```
9. 将小说章节的标题和内容存储到一个Pandas DataFrame中:
```python
novel_df = pd.DataFrame({'title': chapter_titles, 'content': chapter_content})
```
现在,你已经成功地爬取了晋江文学网上的小说数据,并将其存储在一个DataFrame中。可以使用Pandas的导出功能将数据导出为CSV或Excel文件,以便进一步分析。
阅读全文
相关推荐
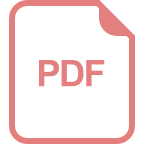
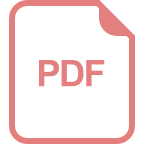













