PTA笛卡尔树用C语言完整版代码
时间: 2024-11-05 18:08:07 浏览: 11
pta笛卡尔树,也称为 Patricia trie(PATRICIA Tree 或 radix tree),是一种用于高效存储和查找字符串的数据结构。它结合了字典树(Trie)和二叉查找树(BST)的特点,通过共享节点减少了存储空间,并支持高效的前缀搜索。
下面是一个简单的C语言实现PITRACTA树的示例,注意这只是一个基本框架,实际应用可能需要更复杂的功能如插入、删除和搜索:
```c
#include <stdio.h>
#include <stdlib.h>
typedef struct Node {
char letter;
int value; // 存储关联数据
struct Node *children[26]; // 用于存储每个字母对应的子节点
} Node;
Node* create_node(char c) {
Node* node = (Node*)malloc(sizeof(Node));
node->letter = c;
node->value = 0;
for (int i = 0; i < 26; ++i)
node->children[i] = NULL;
return node;
}
void insert(Node** root, char* word, int value) {
Node* current = *root;
for (char c : word) {
if (!current->children[c - 'a'])
current->children[c - 'a'] = create_node(c);
current = current->children[c - 'a'];
}
current->value = value;
}
// 示例查找函数,可以根据实际情况进行优化
int search(Node* root, char* prefix) {
Node* current = root;
for (char c : prefix) {
if (!current->children[c - 'a'])
return -1; // 表示没有找到匹配的前缀
current = current->children[c - 'a'];
}
return current->value; // 如果找到,返回关联值;否则返回0或未定义
}
int main() {
Node* root = create_node('\0');
// 插入操作...
char* prefix = "patricia";
int value_to_find = 42;
printf("Value associated with '%s': %d\n", prefix, search(root, prefix));
// 删除和遍历等操作...
return 0;
}
```
阅读全文
相关推荐
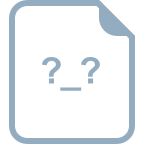
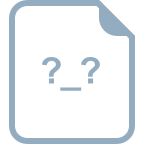








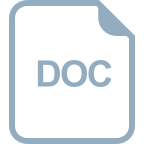






