实现深拷贝val //table复选框 handleSelectionChange(val) { // console.log(1111, val); this.copyList = val },
时间: 2024-10-13 20:14:01 浏览: 29
在JavaScript中,当你有一个需要在组件状态改变时进行深度复制的对象`val`,比如是一个表格数据结构,如数组或对象,并且这个数据可能是复杂的,包含嵌套的对象或数组,你可以通过`JSON.parse()`和`JSON.stringify()`组合的方式来实现浅拷贝。但是,这仅适用于简单的数据结构,对于复杂的数据结构,你需要专门的库,如`lodash`的`_.cloneDeep()`,或者使用递归函数来完成深拷贝。
在这个例子中,你在`handleSelectionChange`方法里想要对`val`进行深拷贝,可以这样做:
```javascript
import { _.cloneDeep } from 'lodash';
handleSelectionChange(val) {
const copiedVal = _.cloneDeep(val); // 使用lodash的deep clone方法进行深拷贝
this.copyList = copiedVal;
}
```
这样,无论原`val`有多深的嵌套,`copyList`都会拥有完全独立的副本,其修改不会影响原始数据。
相关问题
使用Scala 优化以下代码 def copyDirectory(source: File, destination: File): Unit = { if (source.isDirectory) { if (!destination.exists()) { destination.mkdir() } val files = source.listFiles() for (f <- files) { val newFile = new File(destination.getAbsolutePath + File.separator + f.getName) copyDirectory(f, newFile) } } else { val in = new FileInputStream(source) val out = new FileOutputStream(destination) val buffer = new Array[Byte](1024) var length = in.read(buffer) while (length > 0) { out.write(buffer, 0, length) length = in.read(buffer) } in.close() out.close() } }
以下是对 `copyDirectory` 方法进行优化的示例代码:
```scala
import java.io._
object FileCopy {
def main(args: Array[String]): Unit = {
val source = new File("path/to/source/directory")
val destination = new File("path/to/destination/directory")
copyDirectory(source, destination)
}
def copyDirectory(source: File, destination: File): Unit = {
if (!source.exists()) {
return
}
if (source.isDirectory) {
if (!destination.exists()) {
destination.mkdir()
}
val files = Option(source.listFiles()).getOrElse(Array.empty[File])
for (f <- files) {
val newFile = new File(destination, f.getName)
copyDirectory(f, newFile)
}
} else {
val in = new FileInputStream(source)
val out = new FileOutputStream(destination)
try {
val buffer = new Array[Byte](4096)
var bytesRead = in.read(buffer)
while (bytesRead != -1) {
out.write(buffer, 0, bytesRead)
bytesRead = in.read(buffer)
}
} finally {
in.close()
out.close()
}
}
}
}
```
在上述代码中,我们首先添加了一个条件判断,如果源文件或目录不存在,则直接返回。接着,我们使用 `Option` 对 `source.listFiles()` 方法的返回值进行了安全处理,如果为空则返回一个空数组。这样可以避免在遍历文件时出现空指针异常。另外,我们使用了 `File` 的构造函数来创建新文件对象,而不是使用字符串拼接的方式。这样可以提高代码的可读性。
在文件复制的部分,我们使用了 try-finally 语句块来确保输入和输出流都能被正确地关闭。此外,我们将缓冲区的大小增加到了 4096 字节,这样可以提高文件复制的效率。
为每句代码做注释:for class_name in class_names: current_class_data_path = os.path.join(src_data_folder, class_name) current_all_data = os.listdir(current_class_data_path) current_data_length = len(current_all_data) current_data_index_list = list(range(current_data_length)) random.shuffle(current_data_index_list) train_folder = os.path.join(os.path.join(target_data_folder, 'train'), class_name) val_folder = os.path.join(os.path.join(target_data_folder, 'val'), class_name) test_folder = os.path.join(os.path.join(target_data_folder, 'test'), class_name) train_stop_flag = current_data_length * train_scale val_stop_flag = current_data_length * (train_scale + val_scale) current_idx = 0 train_num = 0 val_num = 0 test_num = 0 for i in current_data_index_list: src_img_path = os.path.join(current_class_data_path, current_all_data[i]) if current_idx <= train_stop_flag: copy2(src_img_path, train_folder) train_num = train_num + 1 elif (current_idx > train_stop_flag) and (current_idx <= val_stop_flag): copy2(src_img_path, val_folder) val_num = val_num + 1 else: copy2(src_img_path, test_folder) # print("{}复制到了{}".format(src_img_path, test_folder)) test_num = test_num + 1 current_idx = current_idx + 1
# 循环遍历每个类别的文件夹
for class_name in class_names:
# 拼接当前类别的数据路径
current_class_data_path = os.path.join(src_data_folder, class_name)
# 获取当前类别的所有数据文件名
current_all_data = os.listdir(current_class_data_path)
# 获取当前类别的数据数量
current_data_length = len(current_all_data)
# 生成当前类别数据的索引列表
current_data_index_list = list(range(current_data_length))
# 随机打乱当前类别数据的索引列表
random.shuffle(current_data_index_list)
# 拼接训练集、验证集、测试集的路径
train_folder = os.path.join(os.path.join(target_data_folder, 'train'), class_name)
val_folder = os.path.join(os.path.join(target_data_folder, 'val'), class_name)
test_folder = os.path.join(os.path.join(target_data_folder, 'test'), class_name)
# 计算训练集、验证集、测试集在当前类别中的截止点
train_stop_flag = current_data_length * train_scale
val_stop_flag = current_data_length * (train_scale + val_scale)
# 初始化当前类别的数据索引、训练集数量、验证集数量、测试集数量
current_idx = 0
train_num = 0
val_num = 0
test_num = 0
# 循环遍历当前类别的数据索引列表,将数据复制到对应的训练集、验证集、测试集文件夹中
for i in current_data_index_list:
src_img_path = os.path.join(current_class_data_path, current_all_data[i])
# 如果当前索引在训练集截止点之前,则将数据复制到训练集
if current_idx <= train_stop_flag:
copy2(src_img_path, train_folder)
train_num = train_num + 1
# 如果当前索引在训练集截止点和验证集截止点之间,则将数据复制到验证集
elif (current_idx > train_stop_flag) and (current_idx <= val_stop_flag):
copy2(src_img_path, val_folder)
val_num = val_num + 1
# 如果当前索引在验证集截止点之后,则将数据复制到测试集
else:
copy2(src_img_path, test_folder)
# 打印当前数据的拷贝信息
# print("{}复制到了{}".format(src_img_path, test_folder))
test_num = test_num + 1
# 更新当前类别的数据索引和数量统计信息
current_idx = current_idx + 1
阅读全文
相关推荐
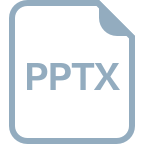
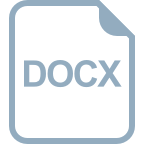
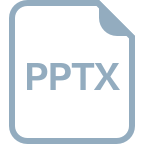
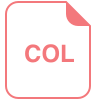













