请写一段完整的Python代码,实现输入DFA输出最小化的DFA
时间: 2024-10-22 18:20:26 浏览: 42
当然可以。在Python中,我们可以使用字典和列表来表示有限状态自动机(DFA),然后通过一些算法来实现最小化过程。这里是一个简单的例子,使用了自顶向下的Kleene算法:
```python
from collections import defaultdict
def create_dfa(states, alphabet, initial_state, transitions):
dfa = {'states': states, 'alphabet': alphabet, 'initial_state': initial_state}
for char in alphabet:
transition_dict = defaultdict(list)
for state in states:
if (state, char) in transitions:
transition_dict[state].append(transitions[(state, char)])
else:
transition_dict[state].append(None)
dfa[char] = transition_dict
return dfa
def minimize_dfa(dfa):
new_states = [dfa['initial_state']]
while True:
added_new_state = False
new_transitions = {}
for char in dfa['alphabet']:
next_states = set()
for current_state in new_states:
for possible_next_state in dfa[char][current_state]:
if possible_next_state is not None and possible_next_state not in new_states:
added_new_state = True
next_states.add(possible_next_state)
if len(next_states) == 1:
next_state = list(next_states)[0]
if char not in new_transitions:
new_transitions[char] = []
new_transitions[char].append(next_state)
else:
if char not in new_transitions:
new_transitions[char] = [{None}]
if not added_new_state:
break
new_states = list(new_transitions.keys()) + list(set().union(*new_transitions.values()))
minimized_dfa = {
'states': new_states,
'alphabet': dfa['alphabet'],
'initial_state': new_states[0],
'transitions': {char: {new_states.index(state): state for state in states} for char, states in new_transitions.items()}
}
return minimized_dfa
# 示例:
states = ['q0', 'q1', 'q2']
alphabet = ['a', 'b']
initial_state = 'q0'
transitions = {
('q0', 'a'): 'q1',
('q0', 'b'): 'q2',
('q1', 'a'): 'q1',
('q1', 'b'): 'q0',
('q2', 'a'): 'q0',
('q2', 'b'): 'q2'
}
dfa = create_dfa(states, alphabet, initial_state, transitions)
minimized_dfa = minimize_dfa(dfa)
print("Minimized DFA:")
for key, value in minimized_dfa.items():
print(f"{key}: {value}")
```
这段代码首先创建了一个基本的DFA结构,然后使用Kleene算法进行最小化。请注意,这只是一个简化的版本,实际应用中可能需要处理更复杂的情况。
阅读全文
相关推荐
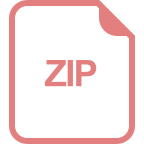
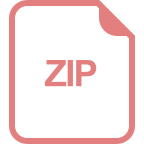
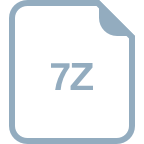











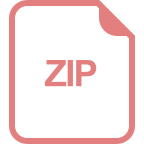
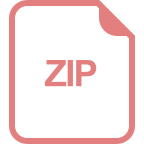
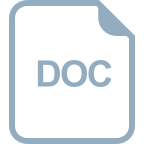
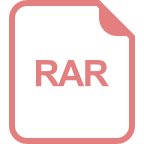
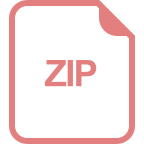