展示字典树的c语言代码
时间: 2024-06-15 14:04:44 浏览: 72
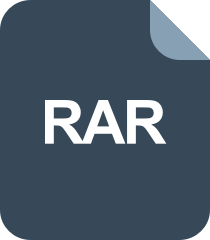
字典树算法 c语言实现

展示字典树的C语言代码如下:
```c
#include <stdio.h>
#include <stdlib.h>
#include <stdbool.h>
#define ALPHABET_SIZE 26
// 字典树的节点结构
struct TrieNode {
struct TrieNode* children[ALPHABET_SIZE];
bool isEndOfWord;
};
// 创建一个新的字典树节点
struct TrieNode* createNode() {
struct TrieNode* node = (struct TrieNode*)malloc(sizeof(struct TrieNode));
node->isEndOfWord = false;
for (int i = 0; i < ALPHABET_SIZE; i++) {
node->children[i] = NULL;
}
return node;
}
// 在字典树中插入一个单词
void insert(struct TrieNode* root, const char* word) {
struct TrieNode* curr = root;
for (int i = 0; word[i] != '\0'; i++) {
int index = word[i] - 'a';
if (curr->children[index] == NULL) {
curr->children[index] = createNode();
}
curr = curr->children[index];
}
curr->isEndOfWord = true;
}
// 在字典树中搜索一个单词
bool search(struct TrieNode* root, const char* word) {
struct TrieNode* curr = root;
for (int i = 0; word[i] != '\0'; i++) {
int index = word[i] - 'a';
if (curr->children[index] == NULL) {
return false;
}
curr = curr->children[index];
}
return (curr != NULL && curr->isEndOfWord);
}
int main() {
// 创建字典树的根节点
struct TrieNode* root = createNode();
// 插入一些单词
insert(root, "apple");
insert(root, "banana");
insert(root, "cat");
insert(root, "dog");
// 搜索单词
printf("%s\n", search(root, "apple") ? "Found" : "Not Found");
printf("%s\n", search(root, "cat") ? "Found" : "Not Found");
printf("%s\n", search(root, "dog") ? "Found" : "Not Found");
printf("%s\n", search(root, "elephant") ? "Found" : "Not Found");
return 0;
}
```
阅读全文
相关推荐
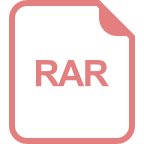
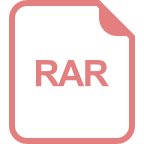
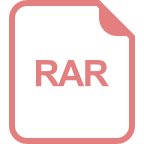
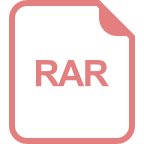
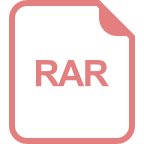
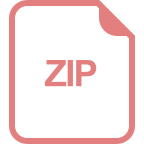
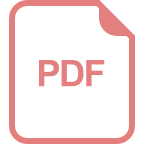
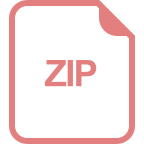
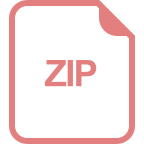
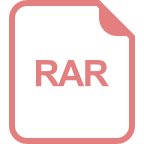
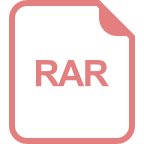
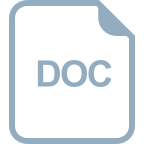
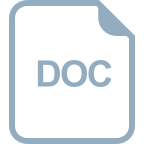
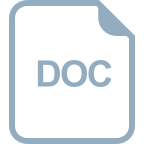
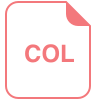
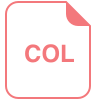