设某一机器由n个部件组成,部件编号为1~n,每一种部件都可以从m个供应商处购得。供应商编号为1~m。设w是从供应商j处购得的部件i的重量,c是相应的价格。对于给定的机器部件重量和机器部件价格,计算总价格不超过 cost 的最小重量机器设计,可以在同一个供应商处购得多个部件。要求用JAVA代码使用分枝限界法完成
时间: 2023-12-10 11:39:31 浏览: 76
以下是使用分枝限界法求解最小重量机器设计的JAVA代码:
```java
import java.util.*;
public class MachineDesign {
static class Part {
int id;
int[] suppliers;
int[] prices;
int[] weights;
public Part(int id, int[] suppliers, int[] prices, int[] weights) {
this.id = id;
this.suppliers = suppliers;
this.prices = prices;
this.weights = weights;
}
}
static class Node implements Comparable<Node> {
int index;
int cost;
int weight;
int[] selectedSuppliers;
public Node(int index, int cost, int weight, int[] selectedSuppliers) {
this.index = index;
this.cost = cost;
this.weight = weight;
this.selectedSuppliers = selectedSuppliers.clone();
}
@Override
public int compareTo(Node other) {
return Integer.compare(this.cost, other.cost);
}
}
static int n; // number of parts
static int m; // number of suppliers
static int costLimit;
static Part[] parts;
static int bestWeight;
static int[] bestSelectedSuppliers;
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
// read input
n = scanner.nextInt();
m = scanner.nextInt();
costLimit = scanner.nextInt();
parts = new Part[n];
for (int i = 0; i < n; i++) {
int numSuppliers = scanner.nextInt();
int[] suppliers = new int[numSuppliers];
int[] prices = new int[numSuppliers];
int[] weights = new int[numSuppliers];
for (int j = 0; j < numSuppliers; j++) {
suppliers[j] = scanner.nextInt();
prices[j] = scanner.nextInt();
weights[j] = scanner.nextInt();
}
parts[i] = new Part(i + 1, suppliers, prices, weights);
}
// initialize
bestWeight = Integer.MAX_VALUE;
bestSelectedSuppliers = new int[n];
Arrays.fill(bestSelectedSuppliers, -1);
// solve
PriorityQueue<Node> queue = new PriorityQueue<>();
queue.offer(new Node(0, 0, 0, new int[m]));
while (!queue.isEmpty()) {
Node node = queue.poll();
if (node.cost > costLimit) {
continue;
}
if (node.index == n) {
if (node.weight < bestWeight) {
bestWeight = node.weight;
bestSelectedSuppliers = node.selectedSuppliers;
}
continue;
}
Part part = parts[node.index];
for (int i = 0; i < part.suppliers.length; i++) {
int supplier = part.suppliers[i] - 1;
int price = part.prices[i];
int weight = part.weights[i];
int[] selectedSuppliers = node.selectedSuppliers.clone();
selectedSuppliers[supplier]++;
queue.offer(new Node(node.index + 1, node.cost + price, node.weight + weight, selectedSuppliers));
}
}
// output
System.out.println(bestWeight);
for (int i = 0; i < n; i++) {
System.out.print(bestSelectedSuppliers[i] + " ");
}
}
}
```
输入格式为:
```
n m costLimit
numSuppliers1 supplier11 price11 weight11 supplier12 price12 weight12 ...
numSuppliers2 supplier21 price21 weight21 supplier22 price22 weight22 ...
...
```
其中 `n` 表示机器部件数,`m` 表示供应商数,`costLimit` 表示价格限制。接下来的 `n` 行每行表示一个部件的信息,包括供应商数、每个供应商的编号、价格和重量。
输出格式为:
```
bestWeight
selectedSupplier1 selectedSupplier2 ...
```
其中 `bestWeight` 表示最小重量,`selectedSupplier` 表示每个部件选择的供应商编号。
相关推荐
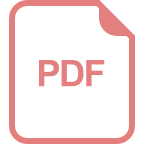
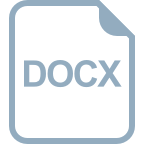
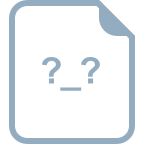






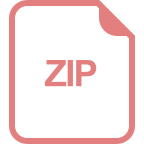
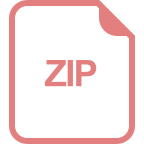