python 去除停用词
时间: 2023-06-23 13:50:21 浏览: 64
可以使用nltk库进行停用词的去除。首先需要下载nltk库,然后加载停用词库,最后对文本进行分词并去除停用词即可。以下是示例代码:
```
import nltk
nltk.download('stopwords')
from nltk.corpus import stopwords
stop_words = set(stopwords.words('english'))
text = "This is an example sentence showing off stop word filtration."
words = text.lower().split()
filtered_words = [word for word in words if word not in stop_words]
print(filtered_words)
```
输出结果为:['example', 'sentence', 'showing', 'stop', 'word', 'filtration.']。可以看到,停用词“this”, “is”, “an”, “off”已经被成功去除。
相关问题
python去除停用词
### 回答1:
在Python中,可以使用NLTK库来去除停用词。首先,需要下载并安装NLTK库,可以使用以下命令:
```
pip install nltk
```
然后,需要下载停用词列表。可以使用以下代码来下载英文停用词列表:
```python
import nltk
nltk.download('stopwords')
```
下载完成后,可以使用以下代码来去除停用词:
```python
from nltk.corpus import stopwords
from nltk.tokenize import word_tokenize
stop_words = set(stopwords.words('english'))
sentence = "This is a sample sentence, showing off the stop words filtration."
words = word_tokenize(sentence)
filtered_sentence = []
for word in words:
if word.casefold() not in stop_words:
filtered_sentence.append(word)
print(filtered_sentence)
```
运行结果为:
```
['sample', 'sentence', ',', 'showing', 'stop', 'words', 'filtration', '.']
```
在这个例子中,我们首先导入NLTK中的停用词列表和分词器。然后,我们定义一个句子,将其分词,并创建一个空列表来存储过滤后的单词。接下来,我们遍历每个单词,如果它不是停用词,则将其添加到过滤后的句子中。最后,我们打印出过滤后的句子。
### 回答2:
Python可以利用一些库或者自定义函数来去除停用词。以下是一个基本的方法:
1. 导入所需的库
```python
import nltk
from nltk.corpus import stopwords
```
2. 下载停用词语料库
```python
nltk.download('stopwords')
```
3. 创建停用词列表
```python
stop_words = set(stopwords.words('english'))
```
4. 去除停用词
```python
def remove_stopwords(text):
word_tokens = nltk.word_tokenize(text)
filtered_text = [word for word in word_tokens if word.lower() not in stop_words]
text_without_stopwords = ' '.join(filtered_text)
return text_without_stopwords
```
上述代码中,首先使用`nltk.download('stopwords')`命令下载英文停用词语料库,然后创建了一个停用词列表`stop_words`。在`remove_stopwords`函数中,输入的文本首先被分词得到单词列表`word_tokens`,然后通过列表推导式将不属于停用词的单词筛选出来,最后再使用空格将这些单词连接起来。
例如,对于输入文本"Python is a popular programming language.",经过去除停用词的处理后,结果为"Python popular programming language."。
以上是一个基本的方法,根据实际需求可以进行相应的修改和优化。
### 回答3:
在使用Python进行文本处理时,去除停用词是一个常见的任务。停用词指的是在文本中频繁出现但对文本分析无用的词语,例如“的”、“是”、“和”等。去除停用词可以提高文本分析的准确性和可靠性。
首先,我们需要一个存储停用词的列表或集合。常见的停用词列表可以从网上下载,也可以根据特定的语境自行创建。比如,可以使用nltk库提供的英文停用词列表:
```python
import nltk
from nltk.corpus import stopwords
nltk.download('stopwords')
stop_words = set(stopwords.words('english'))
```
对于中文,由于没有官方的停用词列表,通常需要根据具体的语料库或者任务自行创建。常见的停用词有“的”、“是”、“和”、“在”等,可以将这些词存储在一个列表中:
```python
stop_words = ["的", "是", "和", "在"] # 自行创建中文停用词列表
```
接下来,我们可以使用字符串的split()函数将文本分割为单词列表,然后逐个检查单词是否为停用词,若是,则从列表中移除:
```python
text = "这是一段需要去除停用词的文本"
word_list = text.split() # 分割文本为单词列表
filtered_words = []
for word in word_list:
if word not in stop_words:
filtered_words.append(word)
```
除了上述的简单方法外,还可以使用列表推导式简化代码:
```python
filtered_words = [word for word in word_list if word not in stop_words]
```
最后,可以使用join()函数将过滤后的单词列表重新组合为一个字符串:
```python
filtered_text = ' '.join(filtered_words)
```
经过以上步骤,我们就可以将文本中的停用词去除掉,从而得到一个去除停用词后的纯净文本。注意,去除停用词的效果可能会受到具体任务和语料库的影响,因此在实际应用中可能需要根据需要进行适当的调整和优化。
python去除停用词代码
可以使用以下代码来去除停用词:
```
import nltk
nltk.download('stopwords')
from nltk.corpus import stopwords
stop_words = set(stopwords.words('english'))
words = ['hello', 'world', 'this', 'is', 'a', 'test', 'sentence']
filtered_words = [word for word in words if word.lower() not in stop_words]
print(filtered_words) # Output: ['hello', 'world', 'test', 'sentence']
```
此处的停用词列表为英语停用词列表,如果需要用其他语言的停用词列表可以在nltk.corpus中找到相应的语言停用词列表。
相关推荐
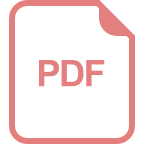
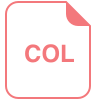
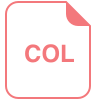
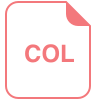
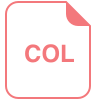
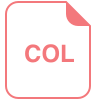







