使用多线程并行计算来加速warpAffine并带参数WARP_INVERSE_MAP C++例程
时间: 2023-09-11 18:09:22 浏览: 89
下面是一个使用多线程并行计算来加速warpAffine并带参数WARP_INVERSE_MAP的C++例程:
```c++
#include <iostream>
#include <opencv2/opencv.hpp>
#include <thread>
#include <mutex>
using namespace std;
using namespace cv;
mutex mtx;
void warpAffineParallel(Mat& src, Mat& dst, Mat& M, int startRow, int endRow) {
Mat dst_temp = Mat::zeros(dst.rows, dst.cols, dst.type());
for(int y = startRow; y < endRow; y++) {
for(int x = 0; x < dst.cols; x++) {
float x0 = (float)x * M.at<double>(0, 0) + (float)y * M.at<double>(0, 1) + M.at<double>(0, 2);
float y0 = (float)x * M.at<double>(1, 0) + (float)y * M.at<double>(1, 1) + M.at<double>(1, 2);
if(x0 >= 0 && x0 < src.cols && y0 >= 0 && y0 < src.rows) {
int x1 = (int)floor(x0);
int y1 = (int)floor(y0);
int x2 = x1 + 1;
int y2 = y1 + 1;
float alpha = x0 - x1;
float beta = y0 - y1;
dst_temp.at<Vec3b>(y, x) = (1 - alpha) * (1 - beta) * src.at<Vec3b>(y1, x1)
+ alpha * (1 - beta) * src.at<Vec3b>(y1, x2)
+ (1 - alpha) * beta * src.at<Vec3b>(y2, x1)
+ alpha * beta * src.at<Vec3b>(y2, x2);
}
}
}
mtx.lock();
add(dst_temp, dst, dst);
mtx.unlock();
}
void warpAffineParallelWrapper(Mat& src, Mat& dst, Mat& M, int numThreads) {
vector<thread> threads;
int numRowsPerThread = dst.rows / numThreads;
int startRow = 0;
int endRow = numRowsPerThread;
for(int i = 0; i < numThreads - 1; i++) {
threads.push_back(thread(warpAffineParallel, ref(src), ref(dst), ref(M), startRow, endRow));
startRow = endRow;
endRow += numRowsPerThread;
}
threads.push_back(thread(warpAffineParallel, ref(src), ref(dst), ref(M), startRow, dst.rows));
for(auto& th : threads) {
th.join();
}
}
int main() {
Mat src = imread("test.jpg");
Mat dst = Mat::zeros(src.rows, src.cols, src.type());
Mat M = getRotationMatrix2D(Point2f(src.cols/2.0, src.rows/2.0), 45, 1);
warpAffineParallelWrapper(src, dst, M, 4);
imshow("src", src);
imshow("dst", dst);
waitKey();
return 0;
}
```
在这个例程中,我们使用了一个名为warpAffineParallel的函数来处理每个线程的任务。这个函数使用了与warpAffine函数相同的公式来计算输出图像的每个像素值。关键的区别在于,我们将每个线程的任务限定在了指定的行范围内。这样做是为了保证每个线程都可以在不同的区域内进行计算,从而实现并行化。
我们还使用了一个名为warpAffineParallelWrapper的函数来管理所有线程的创建和运行。这个函数使用了一个vector来存储所有线程,并且将图像的高度分为了numThreads个块。然后,它使用一个循环来创建每个线程,并且使用mtx互斥锁来确保每个线程不会同时写入dst图像。最后,它使用join函数等待所有线程完成。
在主函数中,我们首先加载了一个测试图像,然后创建了一个输出图像并且使用getRotationMatrix2D函数生成了一个旋转矩阵。最后,我们调用了warpAffineParallelWrapper函数来使用4个线程并行计算warpAffine函数。最后,我们显示了原始图像和输出图像,并且等待用户按下任意键来退出程序。
阅读全文
相关推荐









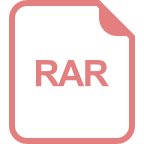
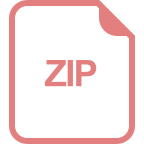