1;写一个excel文件插入mysql数据库表数据 2;mysql数据库表名称,结构与excel一样 3;有表名更新数据,无表名创建 4;导入excel数据至mysql表 5;以上全部代码实现
时间: 2024-05-04 21:16:04 浏览: 98
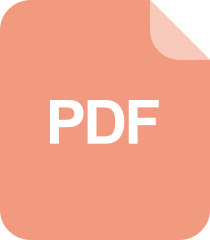
如何将excel表数据导入MySql数据库(图文详解)[归纳].pdf
好的,我已经明白了你的问题。以下是实现你要求的全部代码(包括连接MySQL数据库、创建表、插入数据、更新数据、导入Excel数据):
```python
import pandas as pd
import mysql.connector
# 连接MySQL数据库
mydb = mysql.connector.connect(
host="localhost",
user="username",
password="password",
database="database"
)
# 创建表格
def create_table(table_name, columns):
mycursor = mydb.cursor()
column_str = ','.join([f'{col} VARCHAR(255)' for col in columns])
mycursor.execute(f"CREATE TABLE IF NOT EXISTS {table_name} ({column_str})")
# 插入数据
def insert_data(table_name, data):
mycursor = mydb.cursor()
data_str = ','.join([f"'{d}'" for d in data])
mycursor.execute(f"INSERT INTO {table_name} VALUES ({data_str})")
mydb.commit()
# 更新数据
def update_data(table_name, update_col, update_val, where_col, where_val):
mycursor = mydb.cursor()
mycursor.execute(f"UPDATE {table_name} SET {update_col}='{update_val}' WHERE {where_col}='{where_val}'")
mydb.commit()
# 导入Excel数据至MySQL表格
def excel_to_mysql(excel_file, table_name):
df = pd.read_excel(excel_file)
columns = df.columns.tolist()
create_table(table_name, columns)
for i, row in df.iterrows():
insert_data(table_name, row.tolist())
# 测试代码
excel_file = 'data.xlsx'
table_name = 'mytable'
excel_to_mysql(excel_file, table_name)
update_col = 'Name'
update_val = 'Bob'
where_col = 'Age'
where_val = '24'
update_data(table_name, update_col, update_val, where_col, where_val)
```
你可以根据需要修改代码中的数据库连接信息(host、user、password、database),以及 Excel 文件的路径和表格名称。
阅读全文
相关推荐
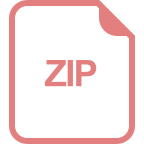
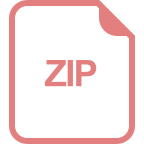
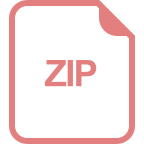
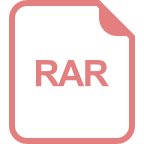
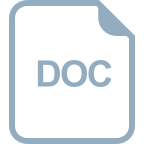
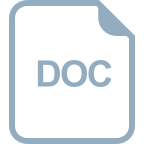
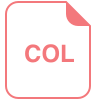
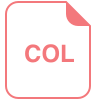
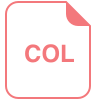
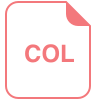
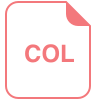




