def extract_coordinates(linestring, vertex_count): coords_str = linestring.replace("LINESTRING(", "").replace(")", "") coords_list = coords_str.split(", ") coords = [(float(coord.split()[0]), float(coord.split()[1])) for coord in coords_list] return coords[:vertex_count]
时间: 2023-06-12 12:06:32 浏览: 174
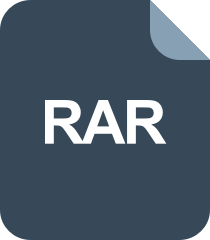
my_regioncoordinates.mat.rar_coordinates.mat_matlab 截取图像_matlab截
这是一个 Python 函数,通过传入一个字符串类型的线串和一个整数类型的顶点数,返回线串中前几个顶点的坐标列表。
具体来说,它首先将线串中的 "LINESTRING(" 和 ")" 字符替换为空字符串,然后用 ", " 将剩余的字符串分割成一个坐标字符串列表。接着,它使用列表推导式将每个坐标字符串转换为一个包含两个浮点数的元组,并将结果存储在一个坐标元组列表中。最后,函数返回前 vertex_count 个坐标元组。
需要注意的是,该函数假定输入的线串是符合标准的 WKT 格式,即以 "LINESTRING(" 开头,以 ")" 结尾,并用 ", " 分隔每个坐标元组。如果输入的线串格式不正确,函数可能会出现异常。
阅读全文
相关推荐
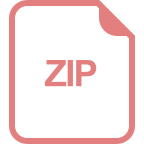
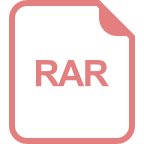












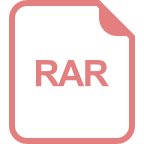
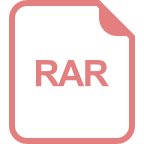
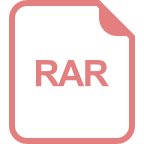
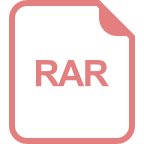