用python语言写一个贪吃蛇小游戏
时间: 2023-05-22 10:03:23 浏览: 57
好的,我可以为您提供关于用 Python 语言编写贪吃蛇小游戏的指导。这需要使用 Python 的图形用户界面库 Pygame,在编码前请确保您已安装 Pygame。以下是一个贪吃蛇游戏的基本结构:
import pygame
import random
pygame.init()
# 设置窗口大小
windowWidth, windowHeight = 600, 400
window = pygame.display.set_mode((windowWidth, windowHeight))
# 设置游戏速度
clock = pygame.time.Clock()
fps = 10
# 定义颜色
white = (255, 255, 255)
black = (0, 0, 0)
red = (255, 0, 0)
green = (0, 255, 0)
blue = (0, 0, 255)
# 初始化贪吃蛇
snakeBlock = 10
snakeSpeed = snakeBlock
snakePos = [windowWidth / 2, windowHeight / 2]
snakeBody = [[snakePos[0], snakePos[1]], [snakePos[0] - snakeBlock, snakePos[1]], [snakePos[0] - (2 * snakeBlock), snakePos[1]]]
# 初始化食物
foodPos = [random.randrange(snakeBlock, windowWidth - snakeBlock, snakeBlock), random.randrange(snakeBlock, windowHeight - snakeBlock, snakeBlock)]
# 定义函数
def gameOver():
font = pygame.font.SysFont(None, 50)
screenText = font.render("Game Over!", True, red)
window.blit(screenText, [windowWidth / 3, windowHeight / 3])
pygame.display.update()
pygame.time.delay(2000)
pygame.quit()
quit()
# 游戏循环
while True:
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
quit()
# 监听键盘事件
keys = pygame.key.get_pressed()
if keys[pygame.K_LEFT]:
snakePos[0] -= snakeSpeed
if keys[pygame.K_RIGHT]:
snakePos[0] += snakeSpeed
if keys[pygame.K_UP]:
snakePos[1] -= snakeSpeed
if keys[pygame.K_DOWN]:
snakePos[1] += snakeSpeed
# 判断贪吃蛇是否吃到食物
if snakePos[0] == foodPos[0] and snakePos[1] == foodPos[1]:
foodPos = [random.randrange(snakeBlock, windowWidth - snakeBlock, snakeBlock), random.randrange(snakeBlock, windowHeight - snakeBlock, snakeBlock)]
else:
snakeBody.pop()
# 判断贪吃蛇是否撞墙或自撞
if snakePos[0] < 0 or snakePos[0] > windowWidth - snakeBlock or snakePos[1] < 0 or snakePos[1] > windowHeight - snakeBlock:
gameOver()
for block in snakeBody:
if snakePos[0] == block[0] and snakePos[1] == block[1]:
gameOver()
# 更新贪吃蛇身体
snakeBody.insert(0, list(snakePos))
# 绘制游戏场景
window.fill(black)
for block in snakeBody:
pygame.draw.rect(window, blue, [block[0], block[1], snakeBlock, snakeBlock])
pygame.draw.rect(window, red, [foodPos[0], foodPos[1], snakeBlock, snakeBlock])
pygame.display.update()
# 控制游戏速度
clock.tick(fps)
# 退出游戏
pygame.quit()
quit()
相关推荐
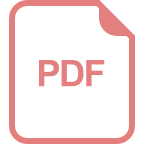
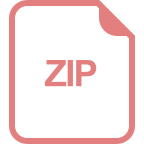
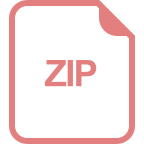














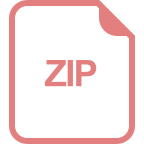