EPSILON = 1e-10 # attention fusion strategy, average based on weight maps # (注意力融合策略,基于权重图的平均值) def attention_fusion_weight(tensor1, tensor2): # avg, max, nuclear (平均、最大值、核) f_spatial = spatial_fusion(tensor1, tensor2) tensor_f = f_spatial return tensor_f # 空间融合 def spatial_fusion(tensor1, tensor2, spatial_type='mean'): shape = tensor1.size() # calculate spatial attention (计算空间注意力) spatial1 = spatial_attention(tensor1, spatial_type) spatial2 = spatial_attention(tensor2, spatial_type) # get weight map, soft-max (获取权重图,soft-max) spatial_w1 = torch.exp(spatial1) / (torch.exp(spatial1) + torch.exp(spatial2) + EPSILON) spatial_w2 = torch.exp(spatial2) / (torch.exp(spatial1) + torch.exp(spatial2) + EPSILON) spatial_w1 = spatial_w1.repeat(1, shape[1], 1, 1) spatial_w2 = spatial_w2.repeat(1, shape[1], 1, 1) tensor_f = spatial_w1 * tensor1 + spatial_w2 * tensor2 return tensor_f # spatial attention # (空间注意) def spatial_attention(tensor, spatial_type='mean'): if spatial_type == 'mean': spatial = tensor.mean(dim=1, keepdim=True) elif spatial_type == 'sum': spatial = tensor.sum(dim=1, keepdim=True) return spatial将上述代码用伪代码表示
时间: 2023-12-06 20:05:11 浏览: 271
对于注意力融合策略,基于权重图的平均值,伪代码如下:
```
EPSILON = 1e-10
# 计算注意力融合权重
def attention_fusion_weight(tensor1, tensor2):
f_spatial = spatial_fusion(tensor1, tensor2)
tensor_f = f_spatial
return tensor_f
# 空间融合
def spatial_fusion(tensor1, tensor2, spatial_type='mean'):
shape = tensor1.size()
# 计算空间注意力
spatial1 = spatial_attention(tensor1, spatial_type)
spatial2 = spatial_attention(tensor2, spatial_type)
# 获取权重图,soft-max
spatial_w1 = torch.exp(spatial1) / (torch.exp(spatial1) + torch.exp(spatial2) + EPSILON)
spatial_w2 = torch.exp(spatial2) / (torch.exp(spatial1) + torch.exp(spatial2) + EPSILON)
spatial_w1 = spatial_w1.repeat(1, shape[1], 1, 1)
spatial_w2 = spatial_w2.repeat(1, shape[1], 1, 1)
tensor_f = spatial_w1 * tensor1 + spatial_w2 * tensor2
return tensor_f
# 空间注意力
def spatial_attention(tensor, spatial_type='mean'):
if spatial_type == 'mean':
spatial = tensor.mean(dim=1, keepdim=True)
elif spatial_type == 'sum':
spatial = tensor.sum(dim=1, keepdim=True)
return spatial
```
阅读全文
相关推荐
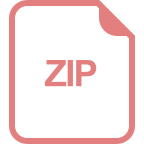
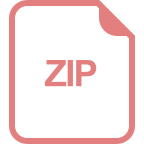
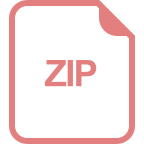
















