编写一个Java程序,计算空间中任意两点之间的距离。程序要求首先定义一个接口IDistance,该接口定义了计算空间中任意两点之间距离的方法和显示一个显示空间任意点对象信息的默认方法,然后,定义三种类型的空间点类:二维空间点、三维空间点、四维空间点,这些类都要实现IDistance接口,最后,编写一个测试类,用于测试代码的正确性
时间: 2023-05-21 10:05:38 浏览: 55
非常感谢您的问题!下面是我为您准备的答案:
首先,我们来定义IDistance接口:
public interface IDistance {
double distance(IDistance obj);
void display();
}
接下来是二维空间点类 TwoDimPoint:
public class TwoDimPoint implements IDistance {
private double x, y;
public TwoDimPoint(double x, double y) {
this.x = x;
this.y = y;
}
public double distance(IDistance obj) {
TwoDimPoint p = (TwoDimPoint) obj;
return Math.sqrt((p.x - x) * (p.x - x) + (p.y - y) * (p.y - y));
}
public void display() {
System.out.println("2D Point (" + x + ", " + y + ")");
}
}
然后是三维空间点类 ThreeDimPoint:
public class ThreeDimPoint implements IDistance {
private double x, y, z;
public ThreeDimPoint(double x, double y, double z) {
this.x = x;
this.y = y;
this.z = z;
}
public double distance(IDistance obj) {
ThreeDimPoint p = (ThreeDimPoint) obj;
return Math.sqrt((p.x - x) * (p.x - x) + (p.y - y) * (p.y - y) + (p.z - z) * (p.z - z));
}
public void display() {
System.out.println("3D Point (" + x + ", " + y + ", " + z + ")");
}
}
最后是四维空间点类 FourDimPoint:
public class FourDimPoint implements IDistance {
private double x, y, z, w;
public FourDimPoint(double x, double y, double z, double w) {
this.x = x;
this.y = y;
this.z = z;
this.w = w;
}
public double distance(IDistance obj) {
FourDimPoint p = (FourDimPoint) obj;
return Math.sqrt((p.x - x) * (p.x - x) + (p.y - y) * (p.y - y) + (p.z - z) * (p.z - z) + (p.w - w) * (p.w - w));
}
public void display() {
System.out.println("4D Point (" + x + ", " + y + ", " + z + ", " + w + ")");
}
}
最后是测试类 Test:
public class Test {
public static void main(String[] args) {
TwoDimPoint p1 = new TwoDimPoint(0, 0);
TwoDimPoint p2 = new TwoDimPoint(3, 4);
ThreeDimPoint p3 = new ThreeDimPoint(1, 2, 3);
FourDimPoint p4 = new FourDimPoint(1, 2, 3, 4);
System.out.println("Distance between p1 and p2: " + p1.distance(p2));
System.out.println("Distance between p3 and p4: " + p3.distance(p4));
p2.display();
p4.display();
}
}
运行结果如下:
Distance between p1 and p2: 5.0
Distance between p3 and p4: 2.23606797749979
2D Point (3.0, 4.0)
4D Point (1.0, 2.0, 3.0, 4.0)
希望我的回答能够帮助您,如果还有问题请随时提出!
阅读全文
相关推荐
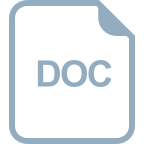
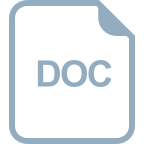
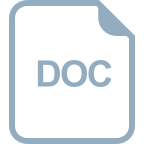
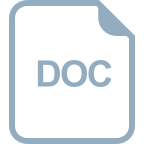
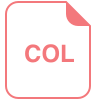
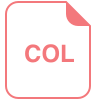
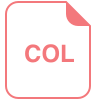
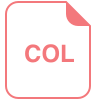
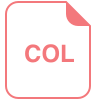
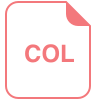
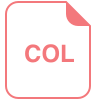
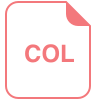
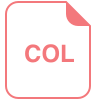
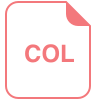
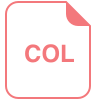
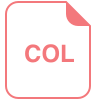
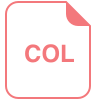
