求任意二叉树中一条最长的路径
时间: 2024-02-24 19:54:36 浏览: 31
任意二叉树中一条最长的路径也被称为直径。求直径的算法是先找到根节点的左子树和右子树的最大深度,然后将两个最大深度相加即可。具体的实现过程如下:
1. 递归地计算左子树和右子树的最大深度,分别为 leftDepth 和 rightDepth。
2. 计算通过根节点的直径长度,即 leftDepth + rightDepth + 1。
3. 在左子树和右子树中递归地查找直径长度最大的路径,并返回其长度。
4. 最后比较通过根节点的直径长度和左右子树中直径长度的最大值,返回最大值即为二叉树的直径长度。
下面是 Python 代码实现:
```python
class TreeNode:
def __init__(self, val=0, left=None, right=None):
self.val = val
self.left = left
self.right = right
class Solution:
def diameterOfBinaryTree(self, root: TreeNode) -> int:
self.ans = 0
def depth(node):
if not node:
return 0
leftDepth = depth(node.left)
rightDepth = depth(node.right)
self.ans = max(self.ans, leftDepth + rightDepth + 1)
return max(leftDepth, rightDepth) + 1
depth(root)
return max(0, self.ans - 1)
```
其中,ans 存储当前找到的最长路径长度,depth 函数递归地计算二叉树的最大深度,并更新 ans 的值。最后,返回最大值即为二叉树的直径长度。
相关推荐
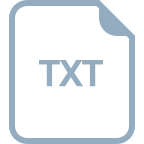
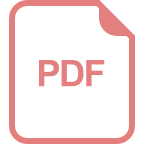








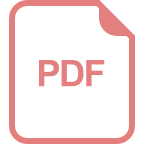
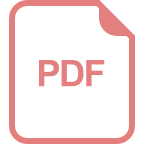
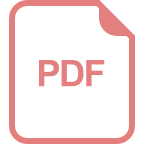
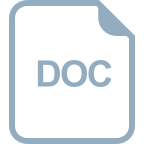
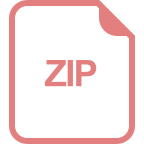
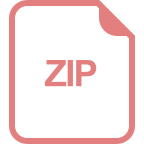
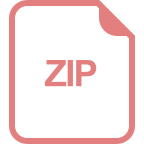