求二分法的比较次数代码
时间: 2024-03-23 09:38:44 浏览: 19
下面是一个求二分查找法比较次数的 Python 代码示例:
```python
def binary_search(arr, x):
low = 0
high = len(arr) - 1
mid = 0
count = 0 # 记录比较次数
while low <= high:
mid = (high + low) // 2
# 如果 x 大于中间元素,则在右侧子数组中查找
if arr[mid] < x:
low = mid + 1
count += 1
# 如果 x 小于中间元素,则在左侧子数组中查找
elif arr[mid] > x:
high = mid - 1
count += 1
# 如果 x 等于中间元素,则返回 mid
else:
count += 1
return count
# 如果未找到 x,则返回 -1
return -1
```
在这个代码中,我们添加了一个 `count` 变量来记录比较次数。每次比较时,我们都将 `count` 加 1。最后,如果找到了元素,则返回 `count`,否则返回 -1。在最坏情况下,二分查找法的比较次数为 `log2(n)`,其中 `n` 是列表中元素的个数。
相关问题
matlab二分法求根代码
二分法求根是一种简单有效的数值计算方法,用于求解非线性方程的根。在Matlab中,可以通过编写函数实现二分法求根。以下是一个简单的例子,演示如何使用二分法求解函数 $f(x) = x^2 - 2$ 在区间 $[1,2]$ 中的根:
```matlab
function [x, n] = bisection_method(f, a, b, tol, max_iter)
% f: 目标函数
% a, b: 区间 [a,b]
% tol: 容差
% max_iter: 最大迭代次数
if f(a)*f(b) >= 0
error('f(a)和f(b)符号相同,无法使用二分法求解');
end
n = 0; % 迭代次数
while (b-a)/2 > tol && n < max_iter
x = (a+b)/2;
if f(x) == 0
return;
elseif f(x)*f(a) < 0
b = x;
else
a = x;
end
n = n+1;
end
x = (a+b)/2;
if n == max_iter
warning('达到最大迭代次数');
end
end
```
使用该函数,可以通过调用以下代码来求解 $f(x) = x^2 - 2$ 在区间 $[1,2]$ 中的根:
```matlab
f = @(x) x^2 - 2;
a = 1;
b = 2;
tol = 1e-6;
max_iter = 100;
[x, n] = bisection_method(f, a, b, tol, max_iter);
fprintf('在区间 [%f,%f] 中,f(x)=0 的根为 x=%f,迭代次数为 %d\n', a, b, x, n);
```
二分法matlab代码
以下是一个二分法的 MATLAB 代码:
```matlab
function [root, fx, ea, iter] = bisect(func, xl, xu, es, maxiter)
% BISECT: root location zeroes
% [root,fx,ea,iter]=bisect(func,xl,xu,es,maxiter,p1,p2,...):
% uses bisection method to find the root of func
% input:
% func = name of function
% xl, xu = lower and upper guesses
% es = desired relative error (default = 0.0001%)
% maxiter = maximum allowable iterations (default = 50)
% output:
% root = real root
% fx = function value at root
% ea = approximate relative error (%)
% iter = number of iterations
if nargin<3,error('at least 3 input arguments required'),end
test = func(xl,varargin{:})*func(xu,varargin{:});
if test>0,error('no sign change'),end
if nargin<4|isempty(es), es=0.0001;end
if nargin<5|isempty(maxiter), maxiter=50;end
iter = 0; xr = xl; ea = 100;
while (1)
xrold = xr;
xr = (xl + xu)/2;
iter = iter + 1;
if xr ~= 0, ea = abs((xr - xrold)/xr) * 100;end
test = func(xl,varargin{:})*func(xr,varargin{:});
if test < 0
xu = xr;
elseif test > 0
xl = xr;
else
ea = 0;
end
if ea <= es | iter >= maxiter, break, end
end
root = xr; fx = func(xr, varargin{:});
```
使用方法:
1. 将以上代码复制到 MATLAB 编辑器中。
2. 在命令行中输入以下命令:
`[root, fx, ea, iter] = bisect(@function_name, xl, xu, es, maxiter)`
- `function_name` 是你要寻找零点的函数名。
- `xl` 和 `xu` 是区间的下限和上限。
- `es` 是相对误差的容许值(默认为 0.0001%)。
- `maxiter` 是最大迭代次数(默认为 50)。
例如:如果你要寻找函数 `f(x) = x^3 - 2*x - 5` 在区间 `[2, 3]` 中的零点,可以输入以下命令:
`[root, fx, ea, iter] = bisect(@func, 2, 3)`
其中 `func` 为一个 M 文件,内容如下:
```matlab
function y = func(x)
y = x^3 - 2*x - 5;
```
运行结束后,`root` 就是所求的零点。
阅读全文
相关推荐




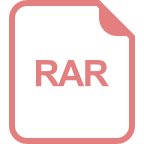








