Linux 系统下的多线程遵循 POSIX 线程接口,称为 pthread。编写 Linux 下的多线程 程序,需要使用头文件 pthread.h,连接时需要使用库 libpthread.a。编写多线程程序 example.c,说明线程创建函数
时间: 2024-05-02 08:17:21 浏览: 12
pthread_create 的使用方法。
pthread_create 函数的原型如下:
```c
int pthread_create(pthread_t *thread, const pthread_attr_t *attr, void *(*start_routine) (void *), void *arg);
```
其中,参数 thread 是一个指向 pthread_t 类型变量的指针,用于存储新创建线程的标识符;参数 attr 是一个指向 pthread_attr_t 类型变量的指针,用于设置线程的属性,可以为 NULL;参数 start_routine 是一个指向函数的指针,用于指定线程要执行的函数;参数 arg 是一个指向 void 类型的指针,用于向线程传递参数。
pthread_create 函数成功时返回 0,失败时返回错误码。在创建线程时,需要使用 malloc 函数动态分配内存来存储参数,并在线程函数执行完毕后使用 free 函数释放内存。
下面是一个简单的例子,展示了 pthread_create 函数的使用方法:
```c
#include <stdio.h>
#include <stdlib.h>
#include <pthread.h>
void *thread_function(void *arg)
{
int i;
printf("Thread function is running. Argument was %d\n", *(int*)arg);
for (i = 0; i < 10; i++) {
printf("Thread function is running. Loop count %d\n", i);
sleep(1);
}
pthread_exit(NULL);
}
int main(void)
{
pthread_t my_thread;
int i;
int arg = 5;
if (pthread_create(&my_thread, NULL, thread_function, &arg)) {
printf("error creating thread.");
abort();
}
for (i = 0; i < 5; i++) {
printf("Main function is running. Loop count %d\n", i);
sleep(1);
}
printf("Waiting for thread to finish...\n");
if (pthread_join(my_thread, NULL)) {
printf("error joining thread.");
abort();
}
printf("Thread joined\n");
return 0;
}
```
在该程序中,main 函数创建了一个新的线程,该线程调用 thread_function 函数,并传递参数 arg。主线程和新线程同时运行,每个线程都打印一些信息。主线程等待新线程结束,然后打印一些信息并退出。
相关推荐
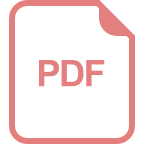
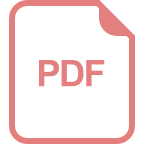














