按先序次序建立一棵二叉树(二叉链表),然后输出树中最小结点值
时间: 2024-02-05 18:02:56 浏览: 78
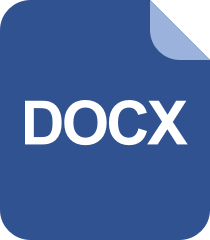
数据结构树练习题.docx
先给出先序遍历二叉树的算法:
1. 访问根结点
2. 先序遍历左子树
3. 先序遍历右子树
根据这个算法,我们可以先建立一棵二叉树:
```python
class TreeNode:
def __init__(self, val):
self.val = val
self.left = None
self.right = None
# 先序遍历建立二叉树
def build_tree():
val = input()
if not val or val == '#':
return None
root = TreeNode(int(val))
root.left = build_tree()
root.right = build_tree()
return root
root = build_tree()
```
建立好二叉树后,我们可以使用中序遍历来找到树中最小结点值。中序遍历的算法是:
1. 中序遍历左子树
2. 访问根结点
3. 中序遍历右子树
由于中序遍历是按照从小到大的顺序访问结点的,所以我们只需要找到第一个被访问的结点即可,即最左边的叶子结点。
```python
# 中序遍历找到最小结点值
def find_min(root):
if not root:
return None
node = root
while node.left:
node = node.left
return node.val
print("树中最小结点值:", find_min(root))
```
完整代码如下:
```python
class TreeNode:
def __init__(self, val):
self.val = val
self.left = None
self.right = None
# 先序遍历建立二叉树
def build_tree():
val = input()
if not val or val == '#':
return None
root = TreeNode(int(val))
root.left = build_tree()
root.right = build_tree()
return root
# 中序遍历找到最小结点值
def find_min(root):
if not root:
return None
node = root
while node.left:
node = node.left
return node.val
root = build_tree()
print("树中最小结点值:", find_min(root))
```
阅读全文
相关推荐
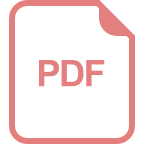
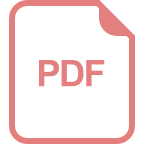





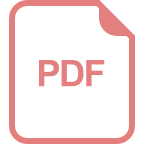
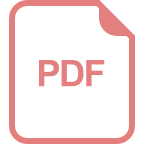
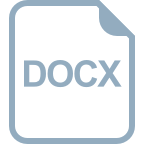
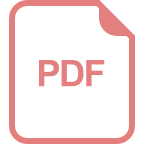
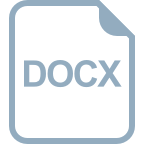
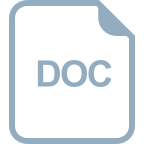
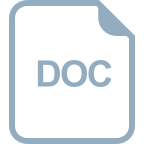