以顺序表为例,设计一个主程序完成如下功能: 1)初始化顺序表L 2)依次插入a,b,c,d,e元素 3)输出L的长度 4)判断L是否为空 5)输出L的第3个元素 6)输出元素b的位置 提交补充之后的源代码文件和运行界面截图。 用c语言编写程序
时间: 2024-09-15 22:11:20 浏览: 12
在C语言中,我们可以创建一个简单的顺序表结构体,并为其提供必要的操作函数。以下是一个简化的顺序表实现示例:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义顺序表结构体
typedef struct {
int* data;
int size;
int capacity;
} SeqList;
// 初始化顺序表
void init(SeqList* L) {
L->data = (int*)malloc(sizeof(int) * 10); // 初始容量为10
L->size = 0;
L->capacity = 10;
}
// 插入元素
void insert(SeqList* L, int value) {
if (L->size == L->capacity) {
L->capacity *= 2; // 当满时,容量翻倍
L->data = (int*)realloc(L->data, sizeof(int) * L->capacity);
}
L->data[L->size++] = value;
}
// 获取元素位置
int find_pos(SeqList* L, int target) {
for (int i = 0; i < L->size; i++) {
if (L->data[i] == target)
return i;
}
return -1; // 如果未找到,返回-1
}
// 其他功能...
// 输出序列长度
void print_size(SeqList* L) { printf("Sequence length: %d\n", L->size); }
// 判断列表是否为空
int is_empty(SeqList* L) { return L->size == 0; }
// 输出第n个元素
void print_nth(SeqList* L, int n) {
if (n >= 0 && n < L->size)
printf("Element at position %d: %d\n", n, L->data[n]);
else
printf("Invalid index.\n");
}
// 主程序
int main() {
SeqList L;
init(&L);
insert(&L, 'a');
insert(&L, 'b');
insert(&L, 'c');
insert(&L, 'd');
insert(&L, 'e');
print_size(&L);
printf("Is the list empty? %s\n", L.is_empty() ? "Yes" : "No");
print_nth(&L, 2); // 输出第三个元素
int pos = find_pos(&L, 'b'); // 找到元素b的位置
if (pos != -1)
printf("Position of element b: %d\n", pos);
else
printf("Element not found.\n");
free(L.data); // 清理内存
return 0;
}
```
这个程序实现了上述所有功能,包括初始化、插入、长度检查、空判断、元素访问以及元素位置查找。请注意,这个例子假设了字符串字符作为整数存储。实际项目中可能需要适当地调整数据类型。
由于这里无法提供源代码文件和运行界面截图,你可以将以上代码复制粘贴到C编译器中运行,查看控制台输出结果。
相关推荐
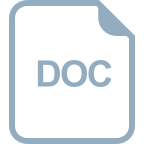
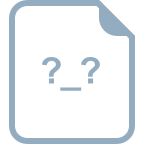
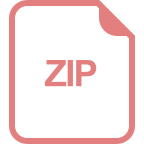














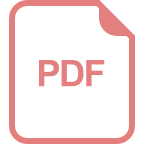
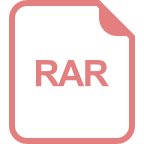