能给我只做一个python贪吃蛇的程序吗
时间: 2023-05-28 15:04:34 浏览: 83
以下是一个简单的Python贪吃蛇游戏程序:
```python
import pygame
import random
# 初始化
pygame.init()
# 游戏窗口大小
WINDOW_WIDTH = 640
WINDOW_HEIGHT = 480
# 蛇身方块大小
BLOCK_SIZE = 20
# 颜色定义
WHITE = (255, 255, 255)
BLACK = (0, 0, 0)
RED = (255, 0, 0)
GREEN = (0, 255, 0)
# 创建游戏窗口
window = pygame.display.set_mode((WINDOW_WIDTH, WINDOW_HEIGHT))
pygame.display.set_caption('贪吃蛇')
# 创建游戏时钟
clock = pygame.time.Clock()
# 定义蛇类
class Snake:
def __init__(self):
self.body = [(WINDOW_WIDTH / 2, WINDOW_HEIGHT / 2)]
self.direction = 'right'
def move(self):
if self.direction == 'right':
new_head = (self.body[0][0] + BLOCK_SIZE, self.body[0][1])
elif self.direction == 'left':
new_head = (self.body[0][0] - BLOCK_SIZE, self.body[0][1])
elif self.direction == 'up':
new_head = (self.body[0][0], self.body[0][1] - BLOCK_SIZE)
elif self.direction == 'down':
new_head = (self.body[0][0], self.body[0][1] + BLOCK_SIZE)
self.body.insert(0, new_head)
self.body.pop()
def draw(self):
for block in self.body:
pygame.draw.rect(window, GREEN, (block[0], block[1], BLOCK_SIZE, BLOCK_SIZE))
def grow(self):
new_tail = self.body[-1]
self.body.append(new_tail)
# 定义食物类
class Food:
def __init__(self):
self.x = random.randint(0, WINDOW_WIDTH - BLOCK_SIZE)
self.y = random.randint(0, WINDOW_HEIGHT - BLOCK_SIZE)
def draw(self):
pygame.draw.rect(window, RED, (self.x, self.y, BLOCK_SIZE, BLOCK_SIZE))
# 创建蛇和食物
snake = Snake()
food = Food()
# 游戏循环
while True:
# 处理游戏事件
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
sys.exit()
elif event.type == pygame.KEYDOWN:
if event.key == pygame.K_RIGHT and snake.direction != 'left':
snake.direction = 'right'
elif event.key == pygame.K_LEFT and snake.direction != 'right':
snake.direction = 'left'
elif event.key == pygame.K_UP and snake.direction != 'down':
snake.direction = 'up'
elif event.key == pygame.K_DOWN and snake.direction != 'up':
snake.direction = 'down'
# 移动蛇
snake.move()
# 判断蛇是否吃到食物
if snake.body[0][0] == food.x and snake.body[0][1] == food.y:
snake.grow()
food = Food()
# 绘制游戏窗口
window.fill(BLACK)
snake.draw()
food.draw()
pygame.display.update()
# 控制游戏帧率
clock.tick(10)
```
这个程序使用Pygame模块实现了一个简单的贪吃蛇游戏,包括蛇的移动、吃食物、增长等功能。你可以根据自己的需要对其进行修改和扩展。
阅读全文
相关推荐
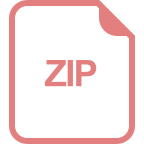
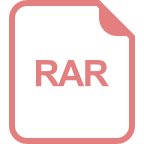










